By: T16-1
Since: Aug 2018
Licence: MIT
- 1. Setting up
- 2. Design
- 3. Implementation
- 4. Documentation
- 5. Testing
- 6. Dev Ops
- 7. Product Scope
- Appendix A: User Stories
- Appendix B: Use Cases
- Appendix C: Non Functional Requirements
- Appendix D: Glossary
- Appendix E: Product Survey
- Appendix F: Instructions for Manual Testing
- F.1. Launch and Shutdown
- F.2. Lock/Unlock
- F.3. Course Management
- F.4. Student Management
- F.5. Module Management
- F.6. Creating a class
- F.7. Listing a class
- F.8. Update Class Enrollment Limits
- F.9. Deleting a class
- F.10. Assign Student To Class
- F.11. Unassign Student From Class
- F.12. Marking class attendance list
- F.13. Access class attendance list
- F.14. Modify class attendance list
- F.15. Gradebook List
- F.16. Gradebook Add
- F.17. Gradebook Find
- F.18. Gradebook Delete
- F.19. Gradebook Edit
- F.20. Grade List
- F.21. Grade Graph
- F.22. Adding a note
- F.23. Deleting notes
- F.24. Editing a note
- F.25. Listing notes
- F.26. Finding notes by keywords
- F.27. Exporting notes to CSV
1. Setting up
1.1. Prerequisites
-
JDK
9
or laterJDK 10
on Windows will fail to run tests in headless mode due to a JavaFX bug. Windows developers are highly recommended to use JDK9
. -
IntelliJ IDE
IntelliJ by default has Gradle and JavaFx plugins installed.
Do not disable them. If you have disabled them, go toFile
>Settings
>Plugins
to re-enable them.
1.2. Setting up the project in your computer
-
Fork this repo, and clone the fork to your computer
-
Open IntelliJ (if you are not in the welcome screen, click
File
>Close Project
to close the existing project dialog first) -
Set up the correct JDK version for Gradle
-
Click
Configure
>Project Defaults
>Project Structure
-
Click
New…
and find the directory of the JDK
-
-
Click
Import Project
-
Locate the
build.gradle
file and select it. ClickOK
-
Click
Open as Project
-
Click
OK
to accept the default settings -
Open a console and run the command
gradlew processResources
(Mac/Linux:./gradlew processResources
). It should finish with theBUILD SUCCESSFUL
message.
This will generate all resources required by the application and tests.
1.3. Verifying the setup
-
Run the
seedu.address.MainApp
and try a few commands -
Run the tests to ensure they all pass.
1.4. Configurations to do before writing code
1.4.1. Configuring the coding style
This project follows oss-generic coding standards. IntelliJ’s default style is mostly compliant with ours but it uses a different import order from ours. To rectify,
-
Go to
File
>Settings…
(Windows/Linux), orIntelliJ IDEA
>Preferences…
(macOS) -
Select
Editor
>Code Style
>Java
-
Click on the
Imports
tab to set the order-
For
Class count to use import with '*'
andNames count to use static import with '*'
: Set to999
to prevent IntelliJ from contracting the import statements -
For
Import Layout
: The order isimport static all other imports
,import java.*
,import javax.*
,import org.*
,import com.*
,import all other imports
. Add a<blank line>
between eachimport
-
Optionally, you can follow the UsingCheckstyle.adoc document to configure Intellij to check style-compliance as you write code.
1.4.2. Updating documentation to match your fork
If you plan to develop this fork as a separate product (i.e. instead of contributing to CS2113-AY1819S1-T16-1/main
), you should do the following:
-
Configure the site-wide documentation settings in
build.gradle
, such as thesite-name
, to suit your own project. -
Replace the URL in the attribute
repoURL
inDeveloperGuide.adoc
andUserGuide.adoc
with the URL of your fork.
1.4.3. Setting up CI
Set up Travis to perform Continuous Integration (CI) for your fork. See UsingTravis.adoc to learn how to set it up.
After setting up Travis, you can optionally set up coverage reporting for your team fork (see UsingCoveralls.adoc).
Coverage reporting could be useful for a team repository that hosts the final version but it is not that useful for your personal fork. |
Optionally, you can set up AppVeyor as a second CI (see UsingAppVeyor.adoc).
Having both Travis and AppVeyor ensures your App works on both Unix-based platforms and Windows-based platforms (Travis is Unix-based and AppVeyor is Windows-based) |
1.4.4. Getting started with coding
When you are ready to start coding,
-
Get some sense of the overall design by reading Section 2.1, “Architecture”.
2. Design
2.1. Architecture
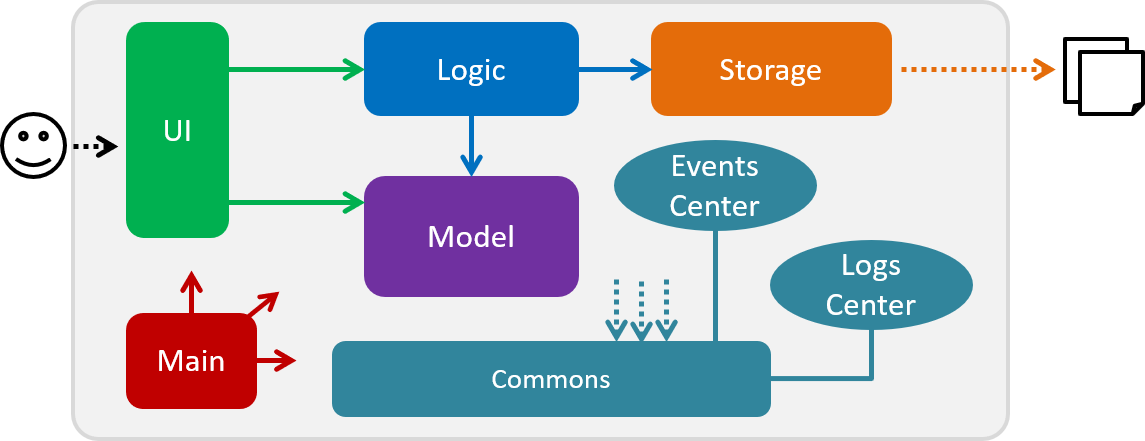
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
The .pptx files used to create diagrams in this document can be found in the diagrams folder. To update a diagram, modify the diagram in the pptx file, select the objects of the diagram, and choose Save as picture .
|
Main
has only one class called MainApp
. It is responsible for,
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components. Two of those classes play important roles at the architecture level.
-
EventsCenter
: This class (written using Google’s Event Bus library) is used by components to communicate with other components using events (i.e. a form of Event Driven design) -
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the class diagram given below) defines its API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
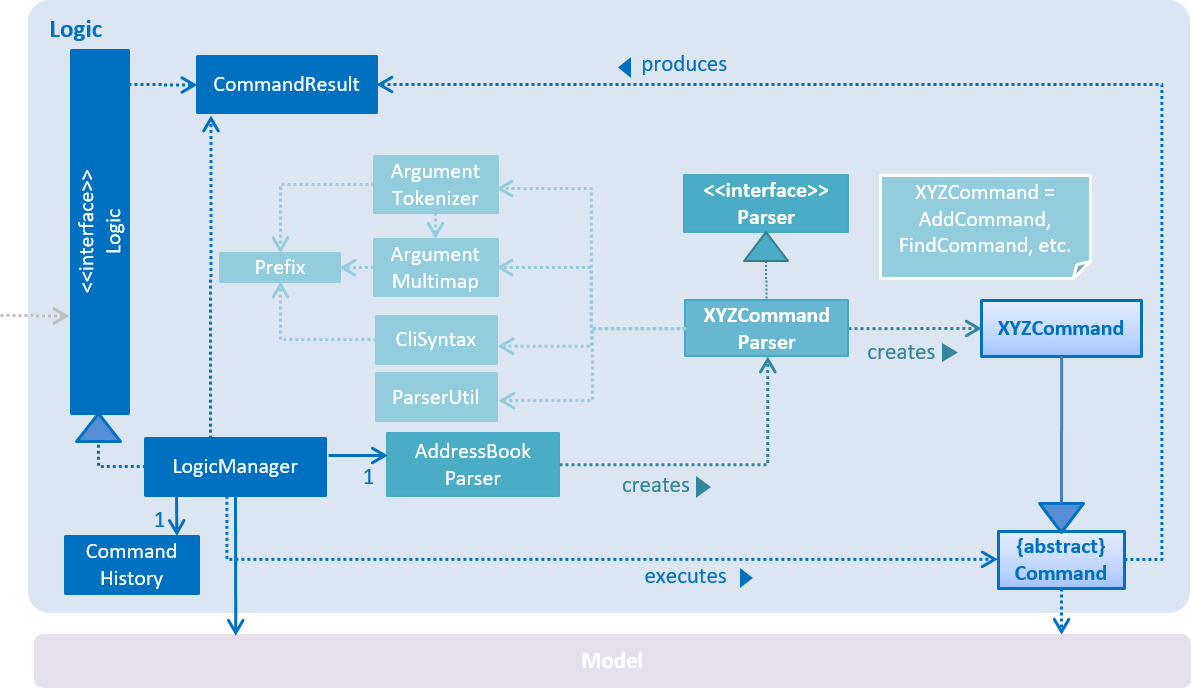
In Trajectory’s implementation, the Model
and Storage
components have been modified slightly to simpler code for the developer team to use. The modifications are explained in greater detail in the sections below.
Events-Driven nature of the design
The Sequence Diagram below shows how the components interact for the scenario where the user issues the command delete 1
.
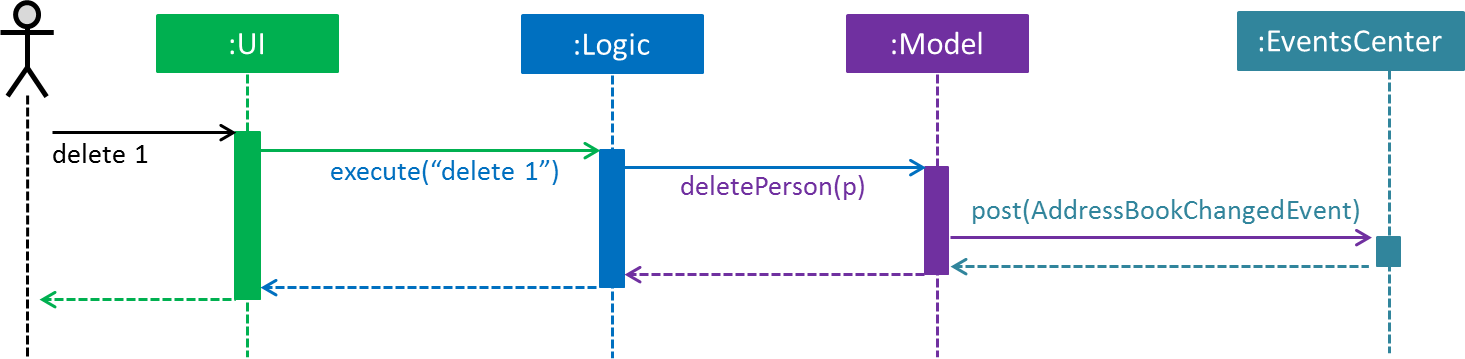
delete 1
command (part 1)
Note how the Model simply raises a AddressBookChangedEvent when the Address Book data are changed, instead of asking the Storage to save the updates to the hard disk.
|
The diagram below shows how the EventsCenter
reacts to that event, which eventually results in the updates being saved to the hard disk and the status bar of the UI being updated to reflect the 'Last Updated' time.
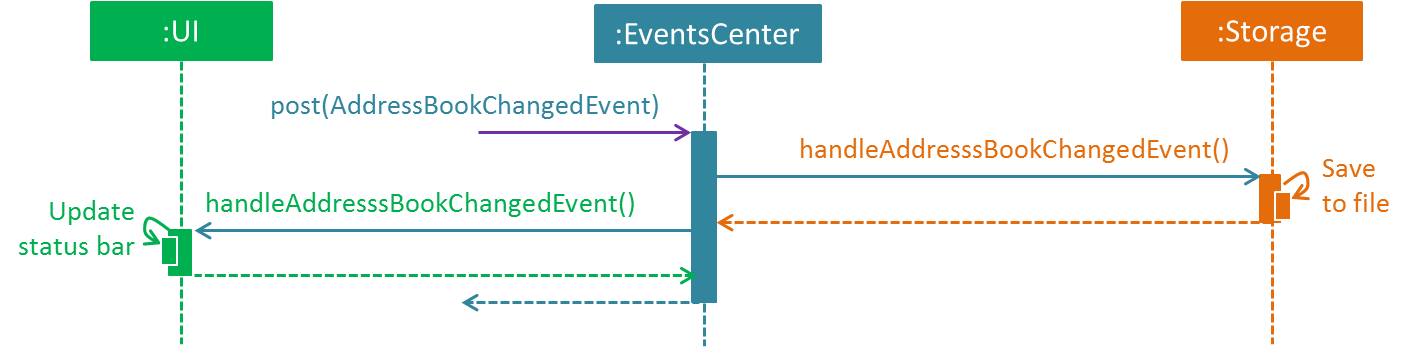
delete 1
command (part 2)
Note how the event is propagated through the EventsCenter to the Storage and UI without Model having to be coupled to either of them. This is an example of how this Event Driven approach helps us reduce direct coupling between components.
|
The sections below give more details of each component.
MVC-inspired design
The event-driven design detailed above was inherited from AddressBook. In Trajectory, a simpler design pattern inspired by the MVC pattern was adopted for the communication between the Model
and Storage
components.
Every new entity in Trajectory exposes its functionality in a {Entity Name}Manager
class. These new manager classes are part of the Model
layer.
On the Storage
layer, a new class StorageController
was added to behave like a proxy for the actual file storage, but without the event-driven design to make things simpler for the team members to add their individual code.
The Sequence Diagram below shows how the Model and Storage components interact in Trajectory’s MVC implementation for the scenario where the user issues the command module delete mc/CS2113
.
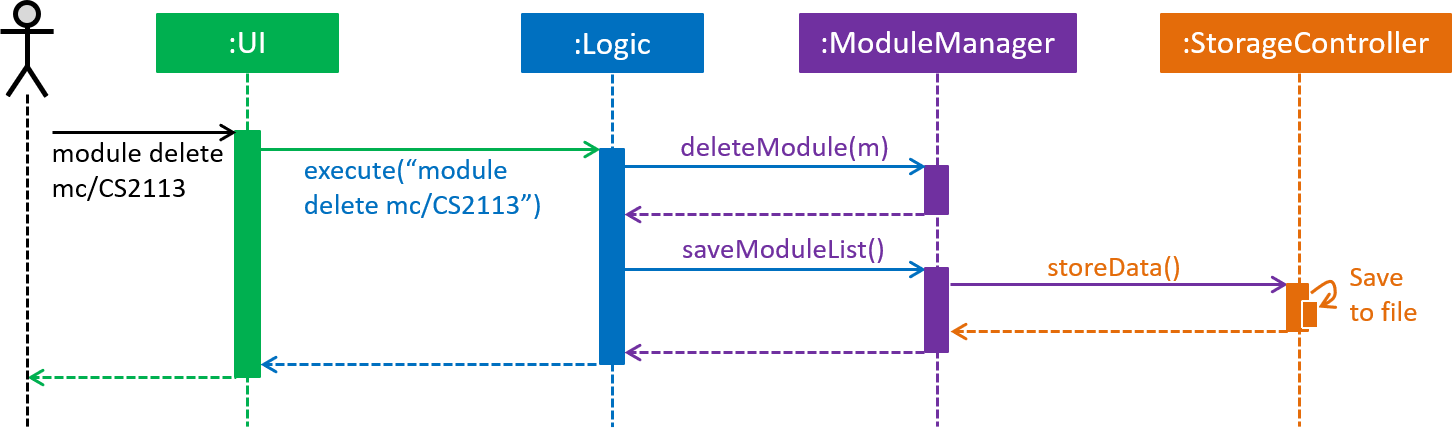
module delete mc/CS2113
command in the MVC implementation
Note how the ModuleManager makes a direct call to StorageController to save the data to the hard disk. This behaviour was partly inspired by the simplicity in Entity Framework’s implementation. It is meant to simplify the storage process and speed up development time.
|
2.2. UI component
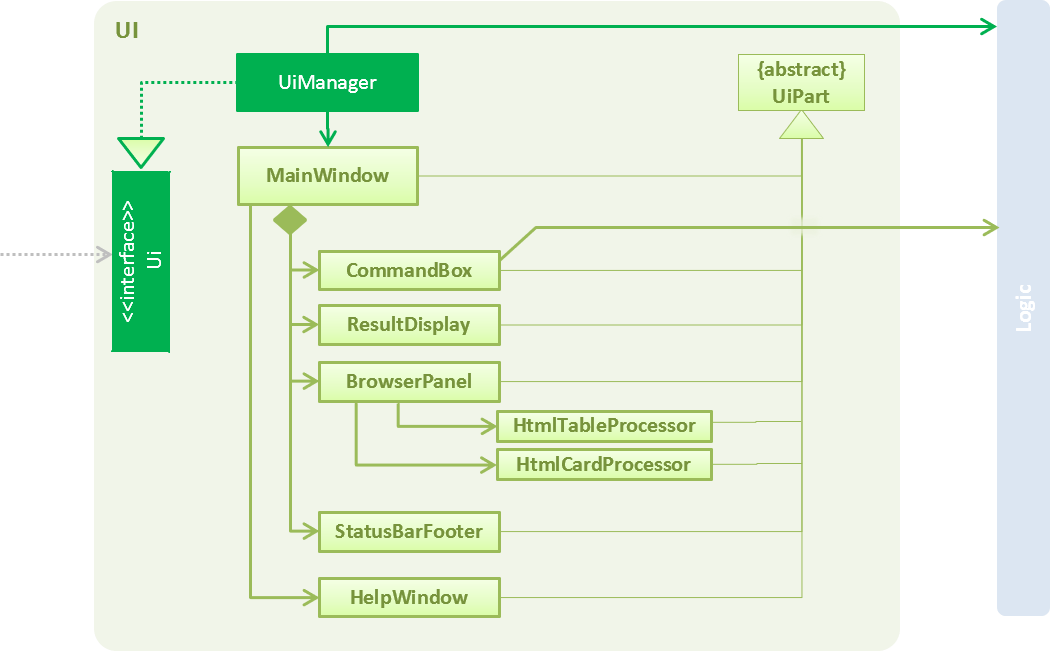
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, StatusBarFooter
, BrowserPanel
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Responds to events raised from various parts of the App and updates the UI accordingly.
-
There are two main components updated in the UI, mainly the ResultDisplay and the BrowserPanel.
-
The BrowserPanel will be updated with either the HtmlTableProcessor or the HtmlCardProcessor.
2.3. Logic component
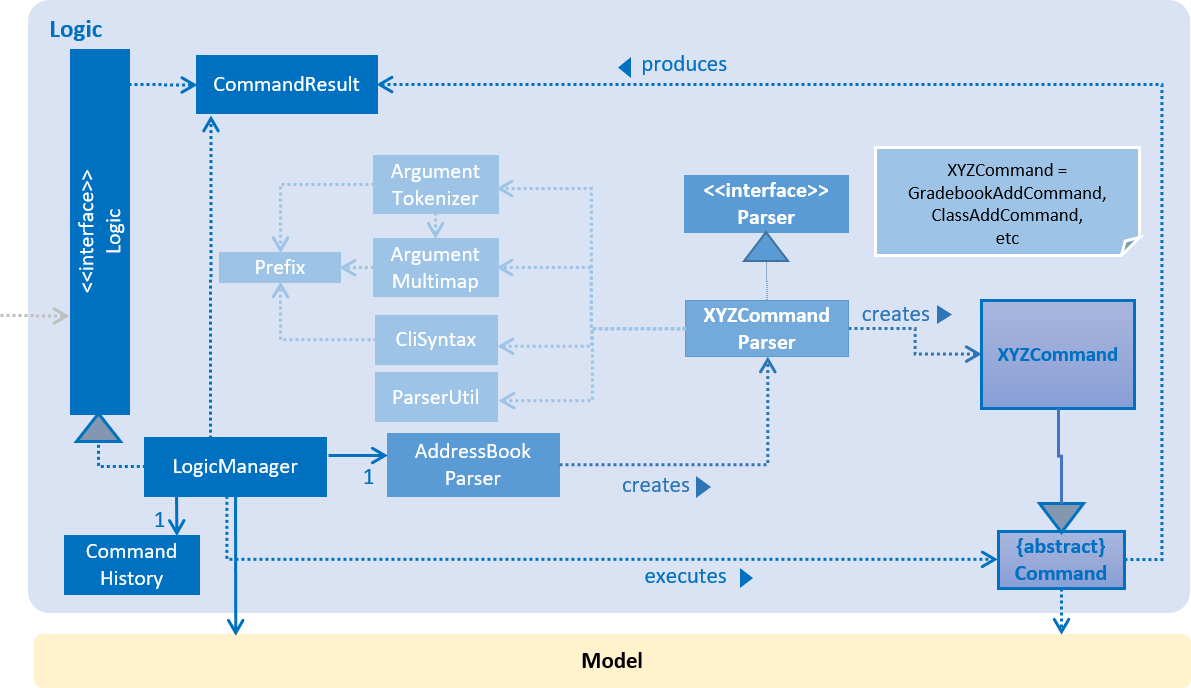
API : Logic.java
-
Logic
uses theAddressBookParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. deleting a gradebook component) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("gradebook delete mc/CS2113 cn/Assignment 1")
API call.
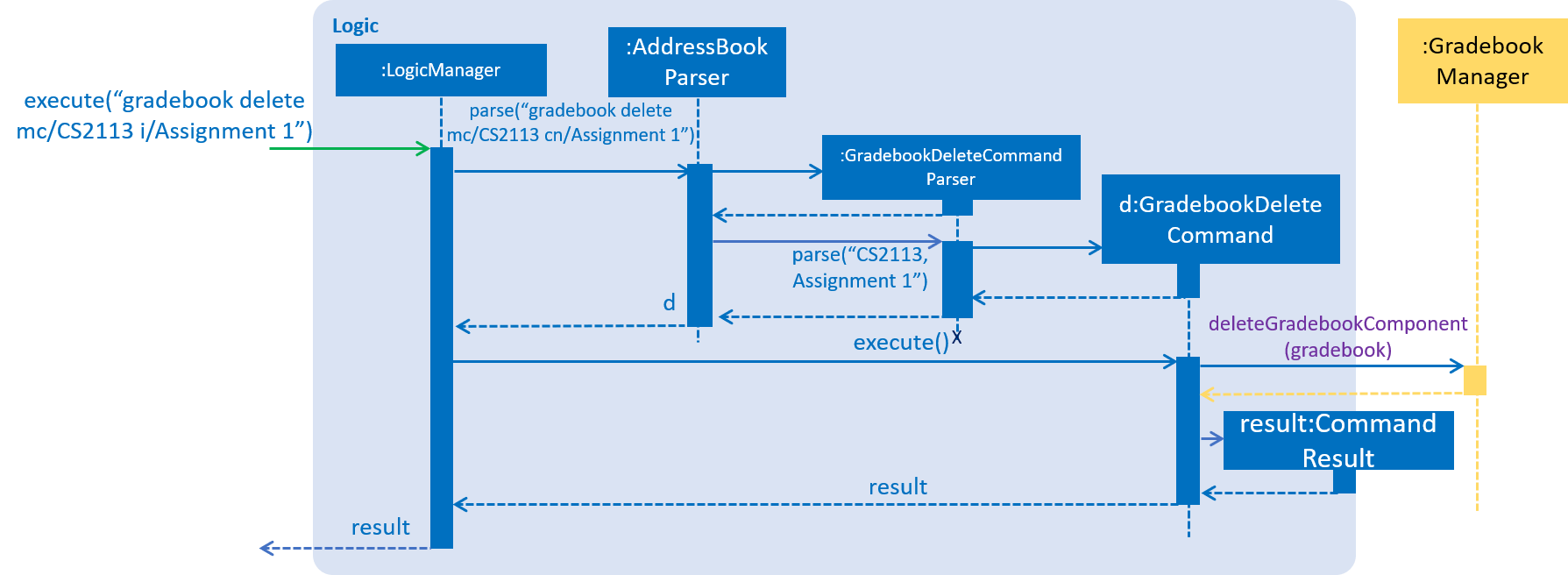
gradebook delete mc/CS2113 cn/Assignment 1
Command2.4. Model component
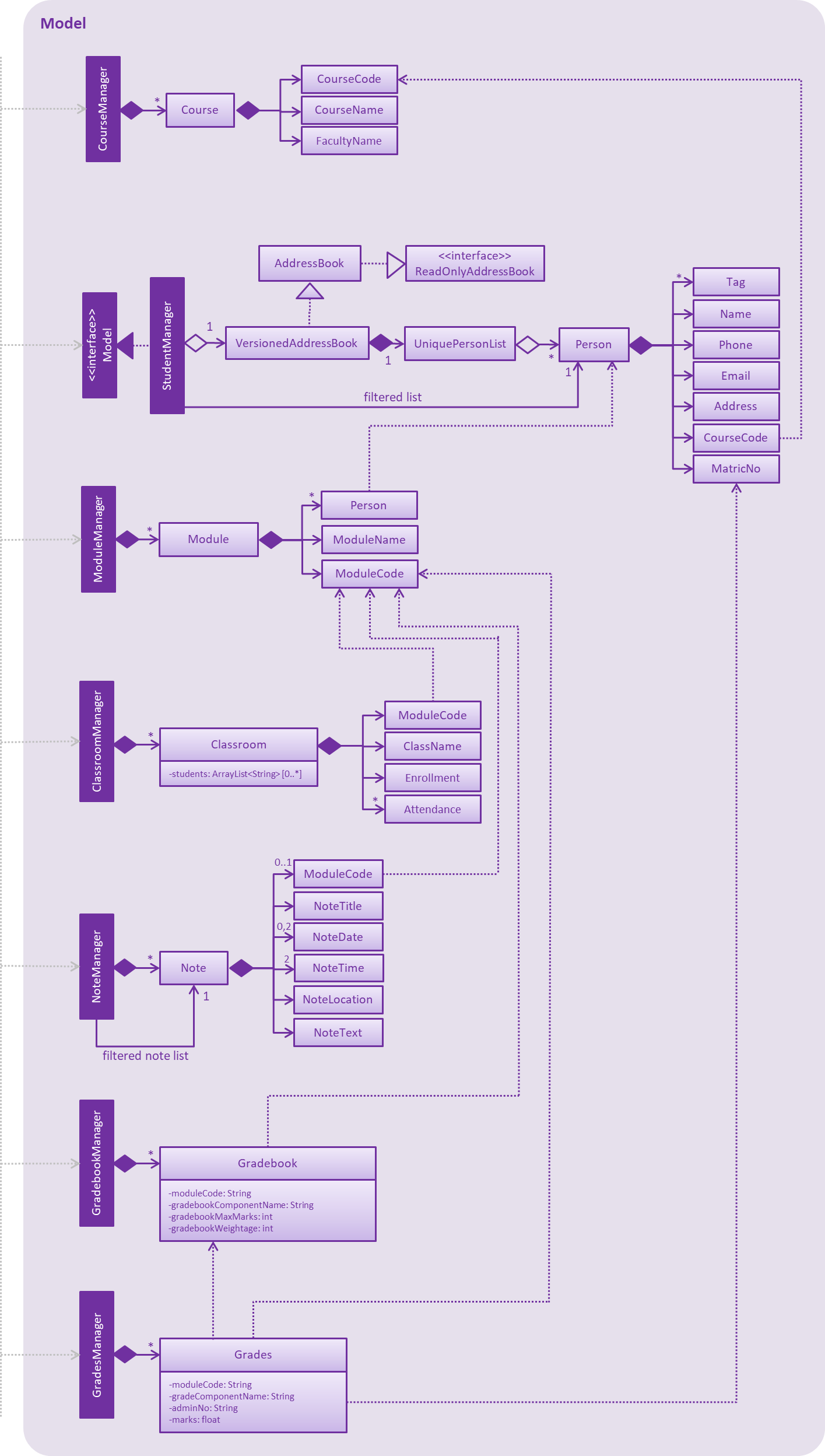
API :
CourseManager.java
Model.java
ModuleManager.java
ClassroomManager.java
GradebookManager.java
GradesManager.java
NoteManager.java
The Model
component is the memory of Trajectory.
It consists of several Manager classes which handles their respective dataset.
The responsibilities of the Model
component are as follows:
-
temporarily holds the user’s data for the duration that the application is running.
-
manages in-memory data changes due to user commands.
-
interacts with the
Storage
component for permanent storage of data to a local storage (e.g. PC’s hard drive). -
exposes a viewable list of data that can be 'observed' by the user. e.g. the UI can render an HTML page that displays the desired information through this list.
Within the Model
component, there are some information dependencies among the different Model Manager classes as shown in Figure 8. Hence, it should be noted that it is not possible to create a data before its parent data due to its hierarchical structure.
2.5. Storage component
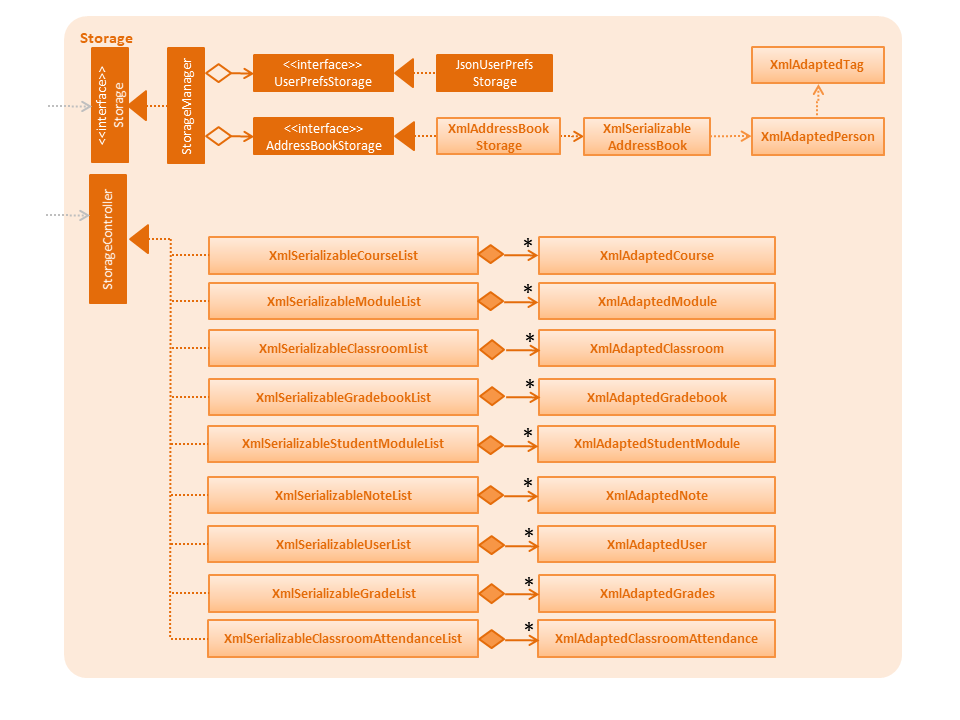
API : Storage.java
The Storage
component consists of two seperate standalone implementations. The base implementation is inherited from AB4 and is solely used for the Student' database. The second implementation, written for Trajectory
is a much more simpler re-imagining of AB4’s code without the levels of abstractions that some might deem excessive. The second implementation is friendlier
for newly-minted developers to use.
For the original built-in AB4’s code, the storage component is capable of
-
can save
UserPref
objects in json format and read it back. -
can save the Address Book data in xml format and read it back.
For the in-house implementation written for Trajectory, the storage component is capable of
-
can save a multitude of different entity data in XML format and read it back.
2.6. Common classes
Classes used by multiple components are in the seedu.addressbook.commons
package.
3. Implementation
This section describes some noteworthy details on how certain features are implemented.
3.1. Undo/Redo feature
3.1.1. Current Implementation
The undo/redo mechanism is facilitated by VersionedAddressBook
.
It extends AddressBook
with an undo/redo history, stored internally as an addressBookStateList
and currentStatePointer
.
Additionally, it implements the following operations:
-
VersionedAddressBook#commit()
— Saves the current address book state in its history. -
VersionedAddressBook#undo()
— Restores the previous address book state from its history. -
VersionedAddressBook#redo()
— Restores a previously undone address book state from its history.
These operations are exposed in the Model
interface as Model#commitAddressBook()
, Model#undoAddressBook()
and Model#redoAddressBook()
respectively.
Given below is an example usage scenario and how the undo/redo mechanism behaves at each step.
Step 1. The user launches the application for the first time. The VersionedAddressBook
will be initialized with the initial address book state, and the currentStatePointer
pointing to that single address book state.

Step 2. The user executes delete 5
command to delete the 5th person in the address book. The delete
command calls Model#commitAddressBook()
, causing the modified state of the address book after the delete 5
command executes to be saved in the addressBookStateList
, and the currentStatePointer
is shifted to the newly inserted address book state.
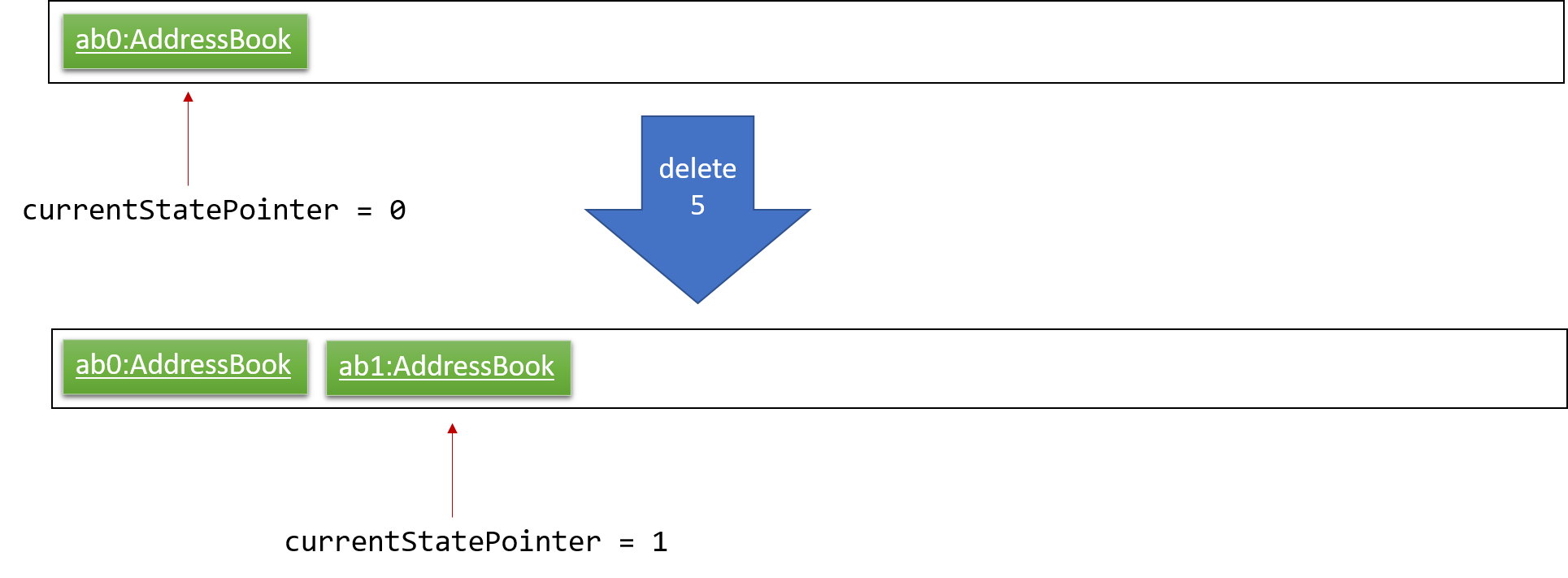
Step 3. The user executes add n/David …
to add a new person. The add
command also calls Model#commitAddressBook()
, causing another modified address book state to be saved into the addressBookStateList
.
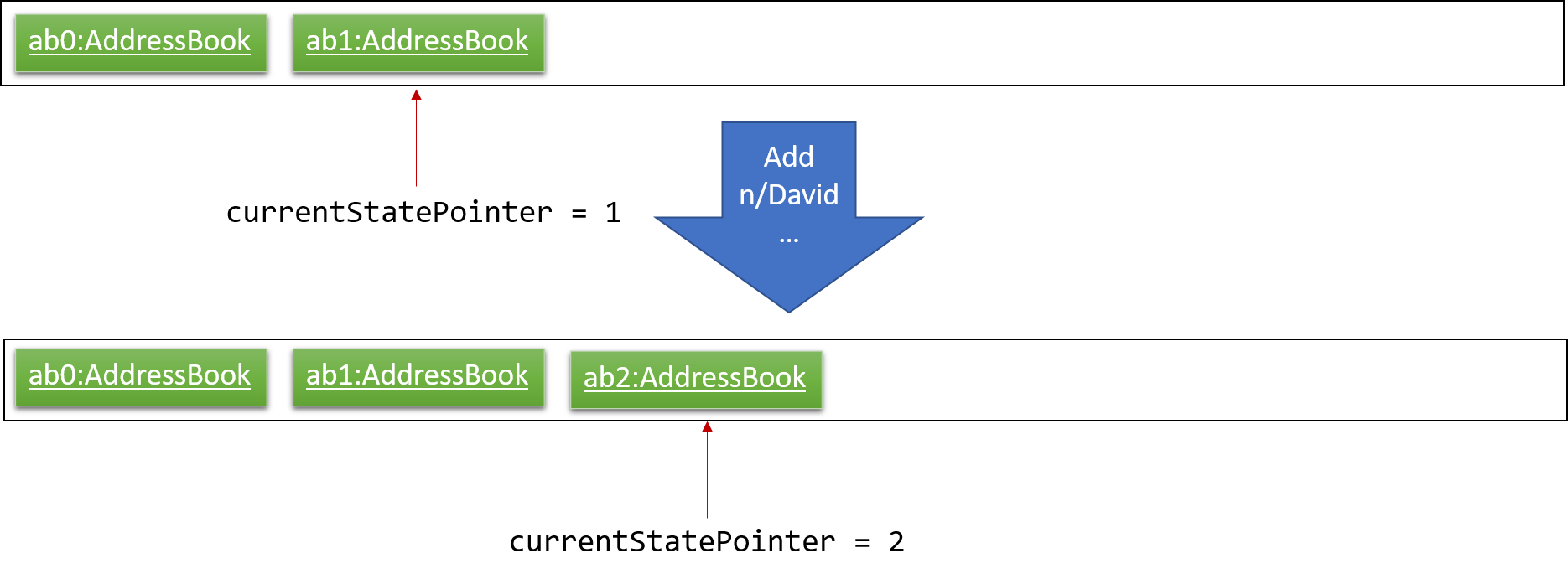
If a command fails its execution, it will not call Model#commitAddressBook() , so the address book state will not be saved into the addressBookStateList .
|
Step 4. The user now decides that adding the person was a mistake, and decides to undo that action by executing the undo
command. The undo
command will call Model#undoAddressBook()
, which will shift the currentStatePointer
once to the left, pointing it to the previous address book state, and restores the address book to that state.
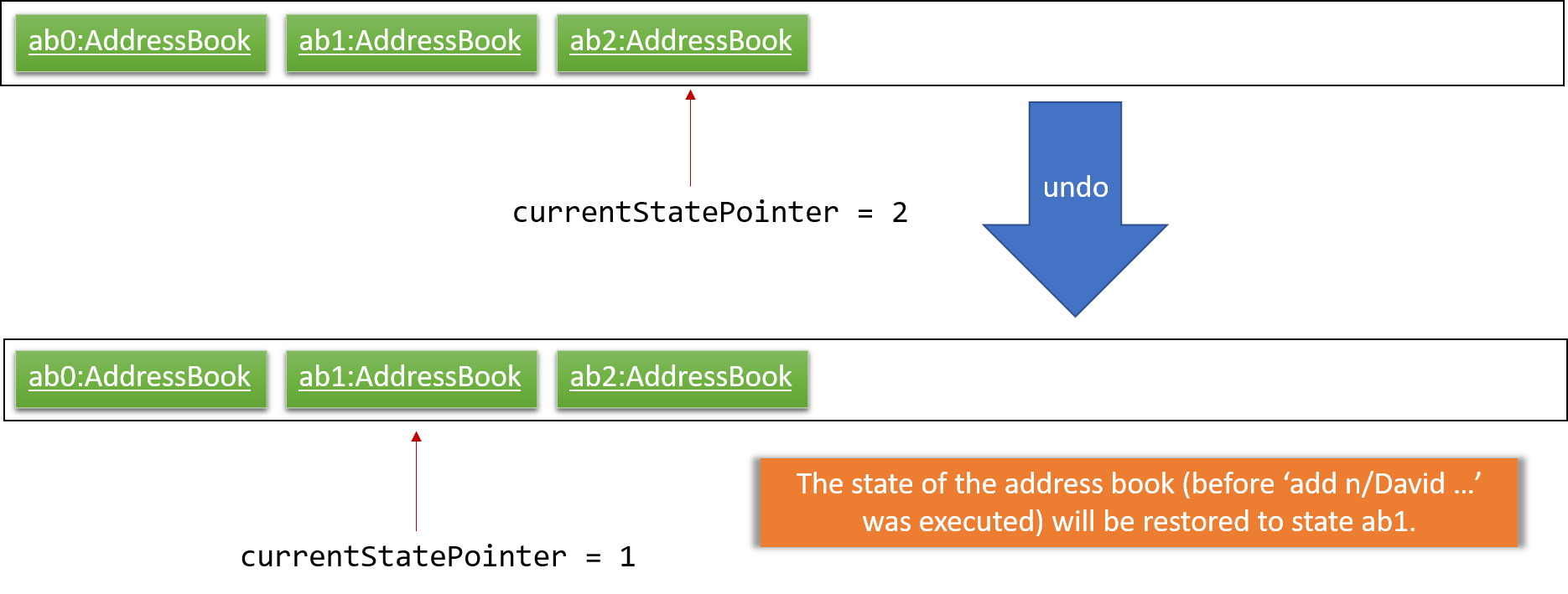
If the currentStatePointer is at index 0, pointing to the initial address book state, then there are no previous address book states to restore. The undo command uses Model#canUndoAddressBook() to check if this is the case. If so, it will return an error to the user rather than attempting to perform the undo.
|
The following sequence diagram shows how the undo operation works:
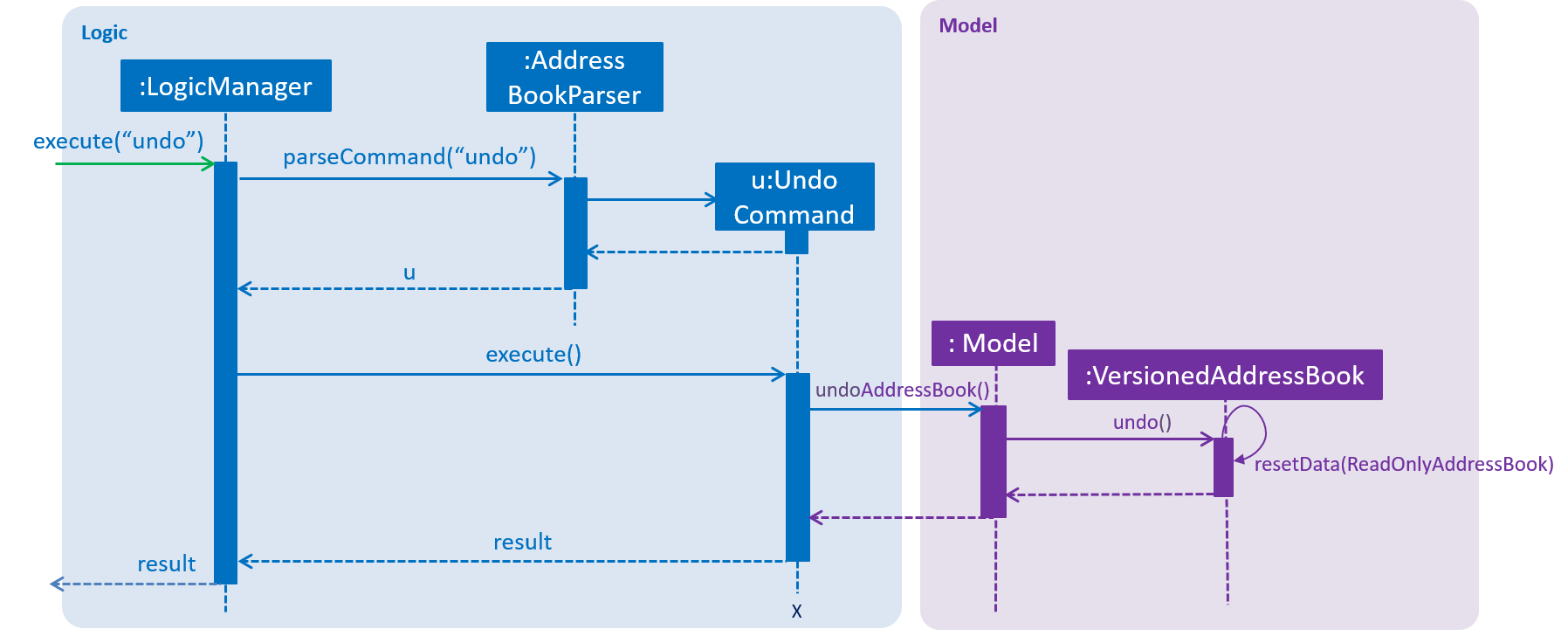
The redo
command does the opposite — it calls Model#redoAddressBook()
, which shifts the currentStatePointer
once to the right, pointing to the previously undone state, and restores the address book to that state.
If the currentStatePointer is at index addressBookStateList.size() - 1 , pointing to the latest address book state, then there are no undone address book states to restore. The redo command uses Model#canRedoAddressBook() to check if this is the case. If so, it will return an error to the user rather than attempting to perform the redo.
|
Step 5. The user then decides to execute the command list
. Commands that do not modify the address book, such as list
, will usually not call Model#commitAddressBook()
, Model#undoAddressBook()
or Model#redoAddressBook()
. Thus, the addressBookStateList
remains unchanged.
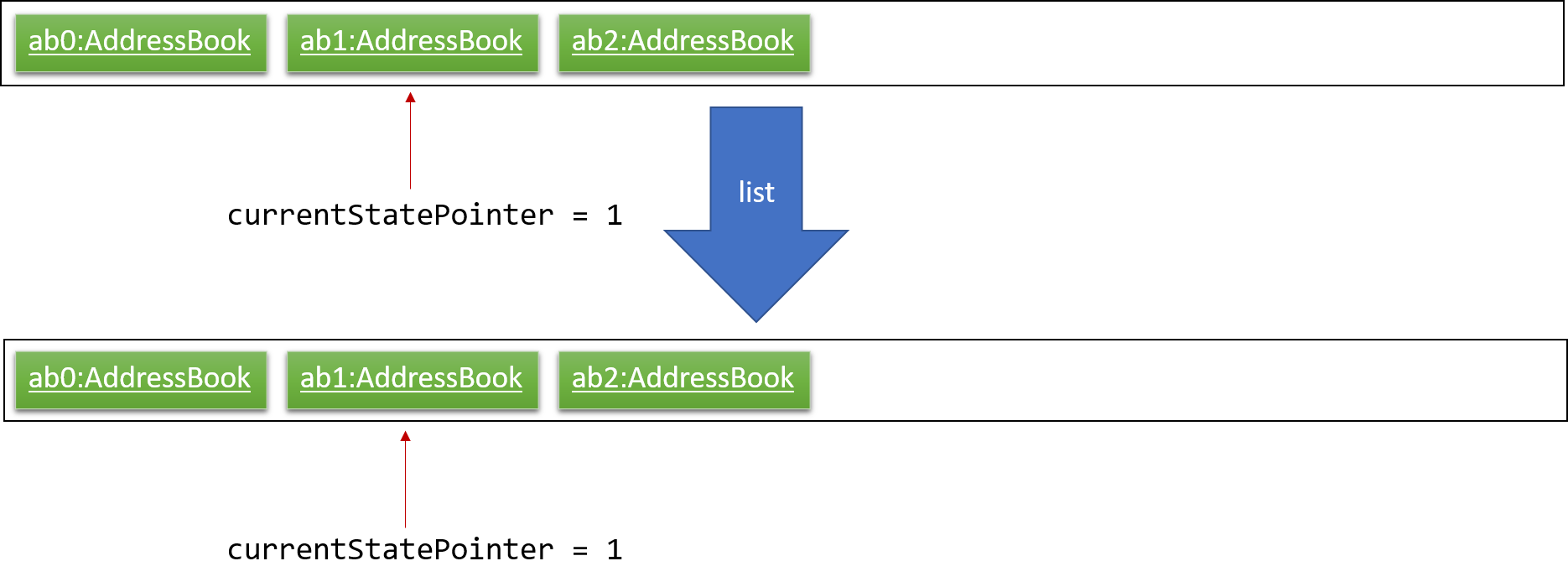
Step 6. The user executes clear
, which calls Model#commitAddressBook()
. Since the currentStatePointer
is not pointing at the end of the addressBookStateList
, all address book states after the currentStatePointer
will be purged. We designed it this way because it no longer makes sense to redo the add n/David …
command. This is the behavior that most modern desktop applications follow.
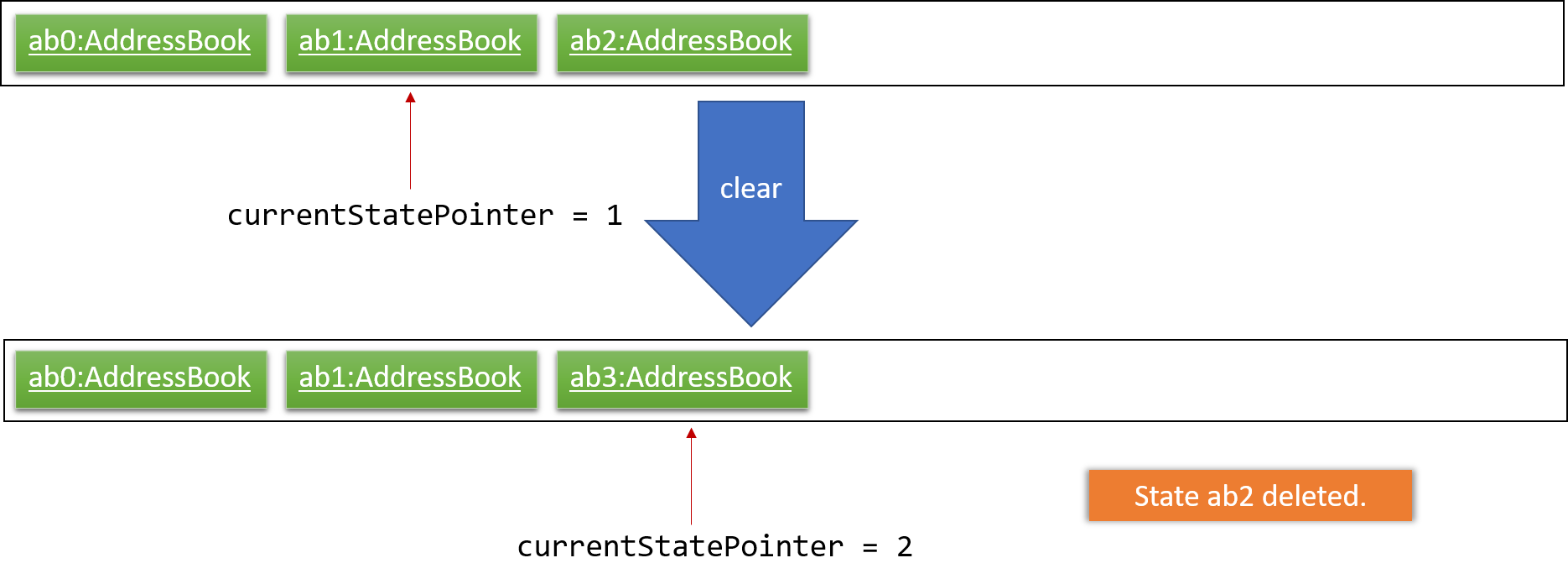
The following activity diagram summarizes what happens when a user executes a new command:
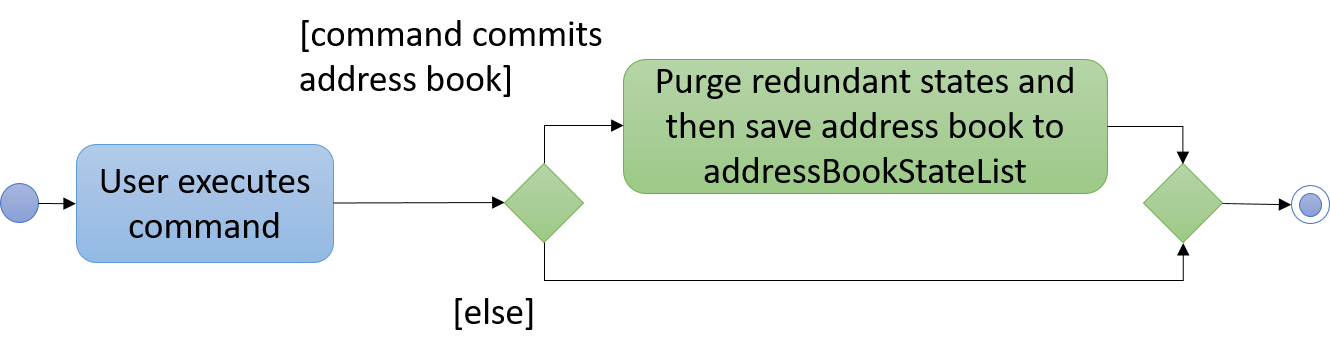
3.1.2. Design Considerations
Aspect: How undo & redo executes
-
Alternative 1 (current choice): Saves the entire address book.
-
Pros: Easy to implement.
-
Cons: May have performance issues in terms of memory usage.
-
-
Alternative 2: Individual command knows how to undo/redo by itself.
-
Pros: Will use less memory (e.g. for
delete
, just save the person being deleted). -
Cons: We must ensure that the implementation of each individual command are correct.
-
Aspect: Data structure to support the undo/redo commands
-
Alternative 1 (current choice): Use a list to store the history of address book states.
-
Pros: Easy for new Computer Science student undergraduates to understand, who are likely to be the new incoming developers of our project.
-
Cons: Logic is duplicated twice. For example, when a new command is executed, we must remember to update both
HistoryManager
andVersionedAddressBook
.
-
-
Alternative 2: Use
HistoryManager
for undo/redo-
Pros: We do not need to maintain a separate list, and just reuse what is already in the codebase.
-
Cons: Requires dealing with commands that have already been undone: We must remember to skip these commands. Violates Single Responsibility Principle and Separation of Concerns as
HistoryManager
now needs to do two different things.
-
3.2. [PROPOSED, PARTIALLY IMPLEMENTED] User Authentication feature
3.2.1. Current Implementation
The bare-bones foundation for user authentication already exists in Trajectory, but it’ll be put in production after V1.4. In the meantime, we have elected to use
elements from that foundation to serve us in a limited capacity. Trajectory is capable of locking/unlocking access to the system via
the unlock
and lock
commands. When the app starts up, the user must type in a password to be able to use the system. Should he decide
to go on a break, he/she need not worry, as the system can be locked just as easily.
This is a sequence diagram of what happens when the user types in the unlock command. Note that you see "Login", as the lock/unlock feature works on top of the user authentication system that already exists in code.
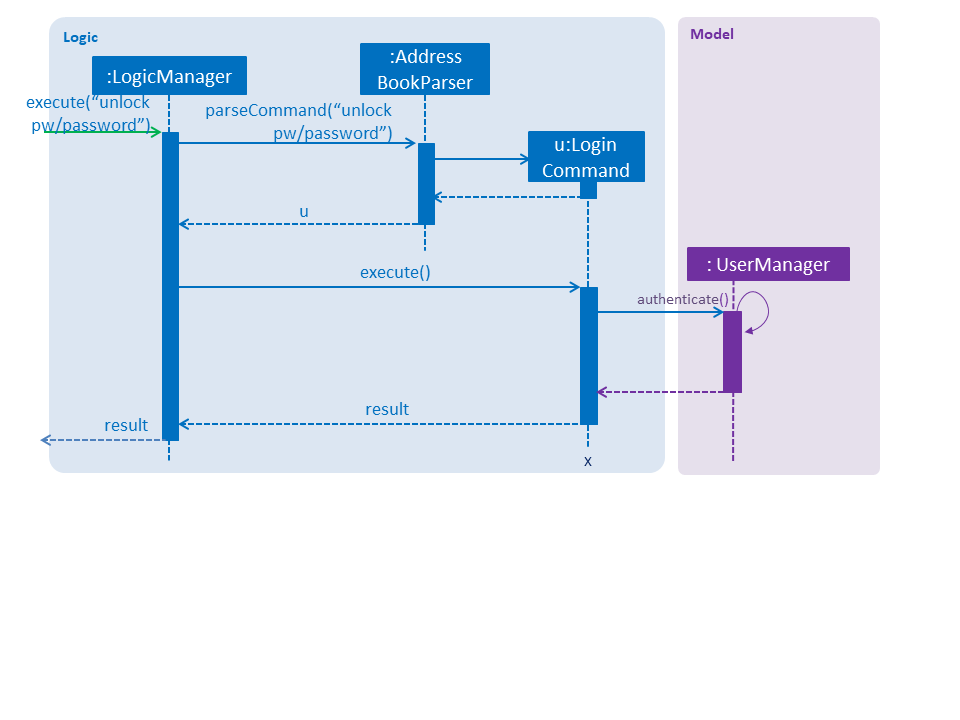
3.2.2. Proposed Implementation
Trajectory will evolve into a full-fledged LMS, and will come equipped with a user authentication system that supports role-based access control for usage by students, faculty members, administrators and guests.
The implementation will be spread across 2 classes: UserController
, AuthenticationController
.
When the user starts up the program, he’ll be prompted to input in his email address, followed by his password. The credentials
are forwarded to AuthenticationController#authenticate()
, whose job is to search for matching credentials in either the 'local storage' or a future DBMS.
The inputted password is hashed, and compared to the saved hash of a matching account, if found. If the password is verified to match, the user is considered to have logged in, and his details
are loaded into the UserController
, which serves as a reference class for other features to access for the details
of the logged-in user, which among other thing includes the user ID, the user personal information and assigned role(s). If the password hash doesn’t match, the user will be shown an error message.
At this point, the user may execute commands. When viewing user-specific data, just as individual modules for students, the relevant controller will access UserController#getLoggedInUserId()
so that
the module controller can appropriately filter out modules that the user has permission to view or access.
This also applies to actions — only faculty members are allowed to create modules, and UserController#getRole()
is queried to check whether the user has that role. An error message will be displayed if the user doesn’t have the required role assigned. Users with the 'administrator' role are allowed to create users, and assign roles to them.
The activity diagram right below is a summary of the login process.
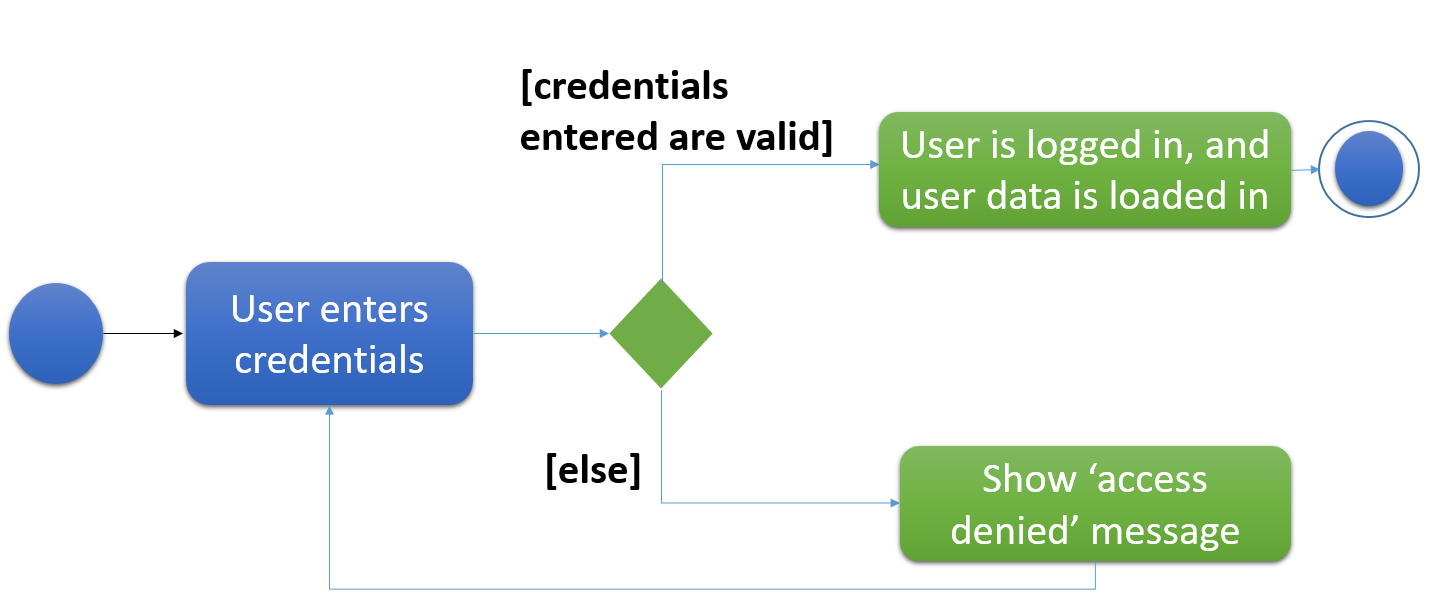
3.2.3. Design Considerations
Aspect: Roles
-
Alternative 1 (current choice): Pre-defined roles with non-changable 'hardcoded' permissions.
-
Pros: Easy to implement.
-
Cons: Limited in expansion. A user with a role cannot do any action belonging to a more powerful role, without being granted the entire set of powers for the higher role.
-
-
Alternative 2: Role-Based Management System
-
Pros: Allows for fine-grained permissions control. Can apply the need-to-know, and need-to-use principle to assign required permissions. For example, the module owner may want to see the gradebook but prevent TAs from seeing it, although TAs can assign marks.
-
Cons: Harder to implement.
-
3.3. Class Add feature
3.3.1. Current Implementation
The add mechanism is facilitated by ClassroomManager
supported by StorageController
.
It makes use of the following operations:
-
ClassroomManager#addClassroom()
— Adds a new classroomList to the in-memory array list. -
ClassroomManager#readClassroomList()
— Gets the classroom list from storage and converts it to a Classroom array list. -
ClassroomManager#saveClassroomList()
— Converts the classroom array list and invokes the StorageController to save the current classroom list to file.
These operations are used in the ClassAddCommand
class under ClassAddCommand#execute()
.
Given below is an example usage scenario and how the add/list mechanism behaves at each step.
Step 1. The user launches the application for the first time. The StorageController#retrieveData()
will retrieve all datasets saved locally.
Step 2. The user executes class add c/16…
command to add a new classroom to Trajectory. The class add
command calls the ClassAddCommand#execute()
. The ClassroomManager
will be instantiated and read the classroom list from the storage and converts the data from XmlAdaptedClassroom to the Classroom data type.
Step 3. The classroomManager#saveClassroomList()
will be called to converts the classroom array list and invokes the StorageController to save the current classroom list to file. This is done by first converting our Classroom
object into XmlAdaptedClassroom
objects and saving it.
The following sequence diagram summarizes what happens when a user executes a new command:
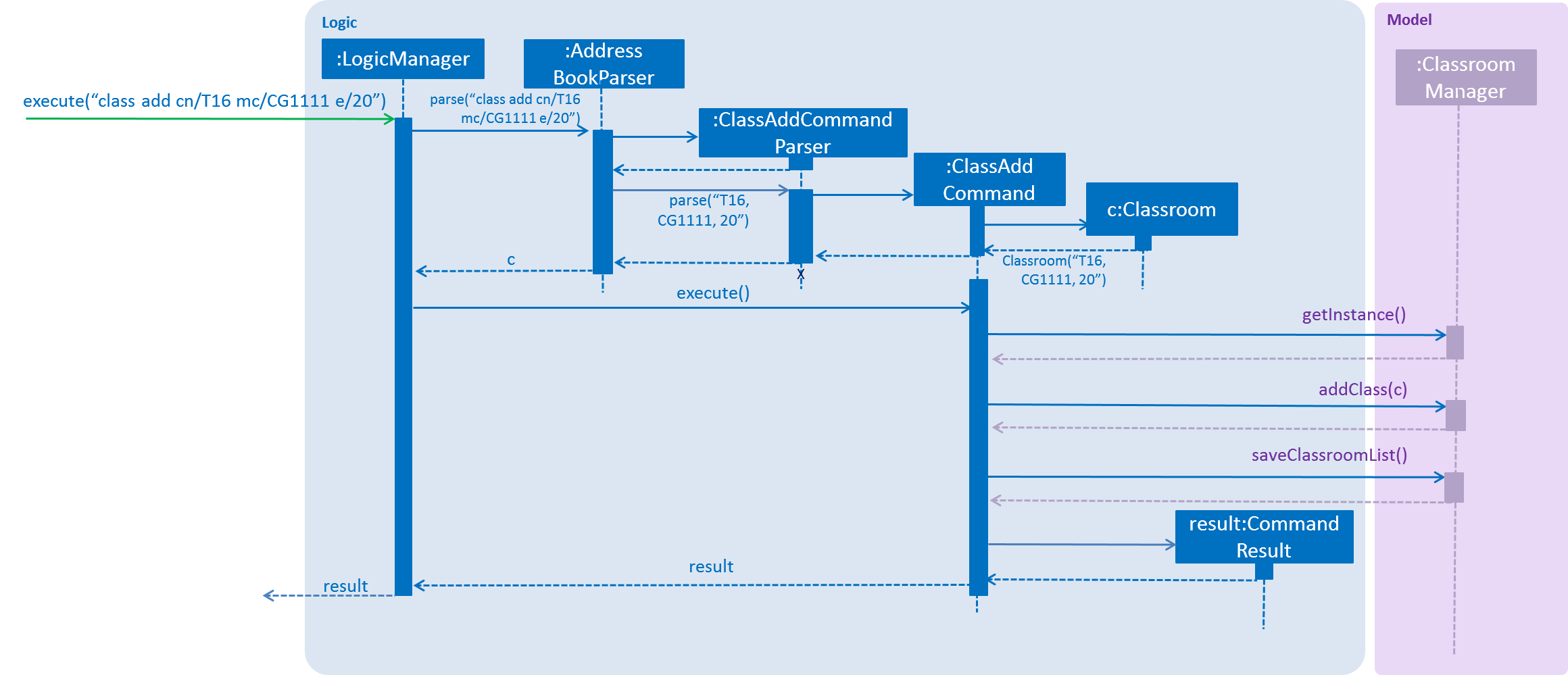
3.4. Class List feature
3.4.1. Current Implementation
The list mechanism is facilitated by ClassroomManager
supported by StorageController
.
It makes use the following operations:
-
ClassroomManager#readClassroomList()
— Gets the classroom list from storage and converts it to a Classroom array list. -
ClassroomManager#saveClassroomList()
— Converts the classroom array list and invokes the StorageController to save the current classroom list to file. -
ClassroomManager#getClassroomList()
— Gets the classroom list from the in-memory array list.
These operations are used in the ClassListCommand
class under ClassListCommand#execute()
..
Given below is an example usage scenario and how the add/list mechanism behaves at each step.
Step 1. The user launches the application for the first time. The StorageController#retrieveData()
will retrieve all datasets saved locally.
Step 2. The user executes class list
command to list all classrooms to Trajectory. The class list
command calls the ClassListCommand#execute()
. The ClassroomManager
will be instantiated and read the classroom list from the storage and converts the data from XmlAdaptedClassroom to the Classroom data type.
Step 3. The classroom list with the corresponding classroom information will be appended to the with the support of the StringBuilder
and displayed as a message successfully.
The following activity diagram summarizes what happens when a user executes a new command:
3.5. Gradebook Graph feature
3.5.1. Current Implementation
The gradebook graph mechanism is an enhancement that will be released in the later versions, facilitated by 'Trajectory'. It is stored internally in GradebookManager.
Additionally, it implements the following operations:
-
gradebookManager#graphModuleSummary()
— Converts data of all student grades from Array List to graph form -
gradebookManager#graphStudentProgress()
— Converts student data to present progress on module.
These operations are exposed in the GradebookManager
as GradebookManager#graphModuleSummary()
, GradebookManager#graphStudentProgress()
respectively.
Given below is an example usage scenario and how the gradebook data-to-graph mechanism behaves at each step.
Step 1. The user launches the application for the first time. The StorageController which interacts with #xmlAdaptedGradebook to retrieve data from Array List using #retrieveData.
Step 2. The user executes gradebook find mc/cs2113 cn/Finals
command to find the relevant gradebook component. The find
command calls GradebookManager#findGradebookComponent()
, which finds and filters the Array List to the relevant search.
Step 3. The user executes gradebook graph student
. GradebookManager#graphStudentProgress will convert the Array List to graph form and display to the user.
If a command fails its execution, it will not call Gradebook#GradebookManager() , so Trajectory state will not be saved into the GradebookManager .
|
Step 4. The user now decides to export graph according to the progress of a student, and that action is done by executing the gradebook graph student
command. This command will call GradebookManager#graphStudentProgress()
, which then displays the graph of the students progress.
The following activity diagram summarizes what happens when a user executes grade graph command:
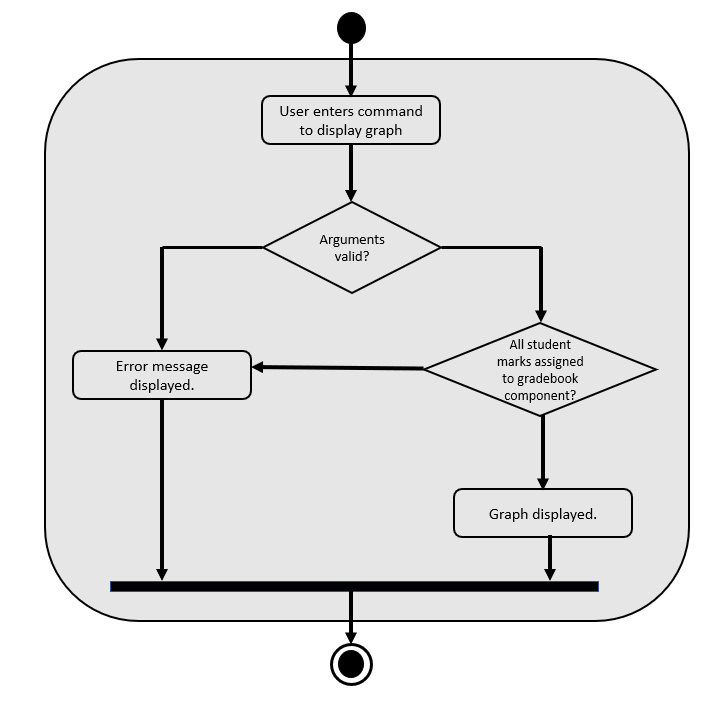
3.5.2. Design Considerations
Aspect: How graph-to-data executes
-
Alternative 1 (current choice): Individual command knows how to export accordingly.
-
Pros: Will use less memory (e.g. only execute command when needed)
-
Cons: Parameters and prefixes must be entered correctly before running command.
-
-
Alternative 2: Saves the entire Trajectory.
-
Pros: Easy to implement.
-
Cons: Might result in low performance due to high memory usage.
-
Aspect: Data structure to support the data-to-graph commands
-
Alternative 1 (current choice): Use a list to store the data before exporting.
-
Pros: Easy data structure to use for any graph.
-
Cons: Large list of data might require significant memory.
-
-
Alternative 2: Use `GradebookManager' for data-to-graph export
-
Pros: We do not need to maintain a separate list, and just reuse what is already in the codebase.
-
Cons: Requires dealing with commands that needs to interact with storage controller or xml adapters directly but command should not have direct interaction from StorageController.
-
3.6. [Proposed] Module bidding feature
3.6.1. Proposed Implementation
The module bidding feature is an enhancement that will make it easier to assign students to modules in Trajectory
.
It is designed with the module enrolment limits in mind, and the aim to give students a certain degree of flexibility
in choosing the modules they want. At the moment, it is planned to store the feature in ModuleManager, but it may be
abstracted into its own class if it proves to be necessary.
The module bidding feature will implement the following features:
-
ModuleManager#startBiddingRound()
— Starts a bidding round for a module. -
ModuleManager#closeBiddingRound()
— Closes the bidding round for a module. -
ModuleManager#placeBid()
— Places a student’s bid on the module they desire. -
ModuleManager#retractBid()
— Retracts a student’s bid from a module for which they previously bid. -
`ModuleManager#assignSuccessfulStudents() — Assigns the students with successful bids to the module.
These operations will be exposed in the ModuleManager
class until there is a need for abstraction.
Given below is an example usage scenario and how the module bidding mechanism will behave at each step.
Step 1. The teacher can start a bidding round for one of his/her modules using the CLI. The command will be routed to
ModuleManager#startBiddingRound()
with the module code to indicate that bidding has opened for that module. This will
also update the status of the module to inform students that they may now start placing bids.
Step 2. A student can place his/her bid for a module with an active bidding round. He/she will need to enter the number
of points they wish to use in their bid. The input will be parsed to ModuleManager#placeBid()
with the module code and
the student’s ID.
Step 3. Should the student decide that he/she is no longer interested in the module, he/she may retract his/her bid by
using the CLI and entering the module’s code. This will invoke ModuleManager#retractBid()
and the bid will be retracted.
Step 4. When the time is past the intended duration of the bidding round, the bidding round can be closed automatically
via a call to ModuleManager#closeBiddingRound()
. When the bidding round has closed, the status will be updated to
reflect it, and students will no longer be able to place bids for the module.
The teacher may close the bidding round earlier by entering the command in the CLI. |
Step 5. After the bidding round has closed, Trajectory will decide which students are successful in their bid by invoking
ModuleManager#assignSuccessfulStudents()
. This will also assign the successful students to the module and deduct their
bid points. The students who were unsuccessful in their bid will have their bid points refunded to their account.
The following activity diagram summarizes the whole module bidding process:
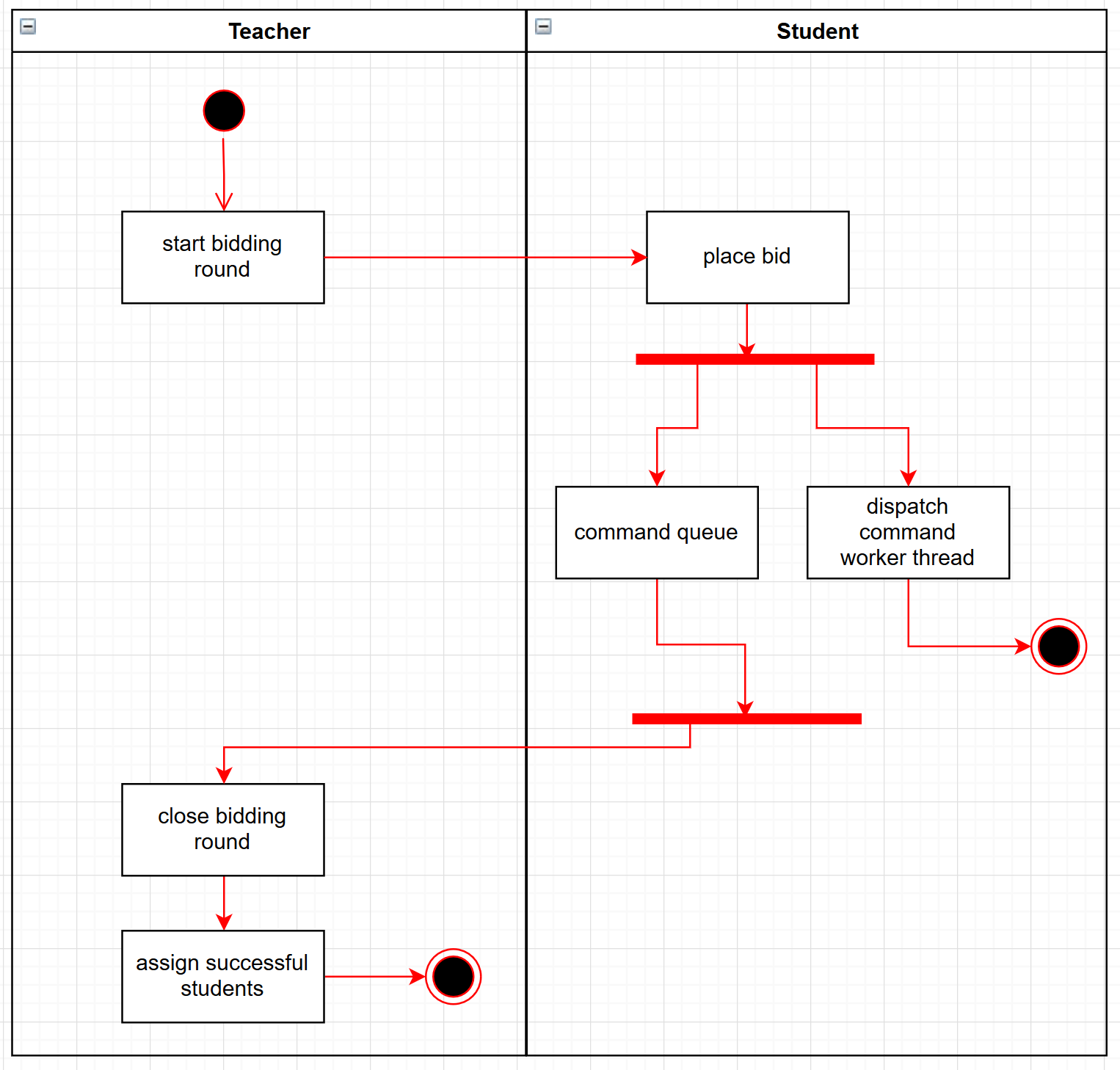
3.6.2. Design Considerations
Aspect: How the module bidding will work
-
Alternative 1 (current choice): Teacher has to manually open a bidding round.
-
Pros: Easy to implement
-
Cons: May result in inconsistencies between the planned start time and the actual start time, thus causing frustration to the students.
-
-
Alternative 2: Teacher can input the start time for a module’s bidding round
-
Pros: The actual start time will be consistent and reliable, leading to user (student) satisfaction.
-
Cons: More difficult to implement; Handling date objects is tricky because there are many popular date formats.
-
3.7. Notes data to Google Calendar feature
3.7.1. Feature Description:
EXPORTING NOTES TO CSV :
The user is able to export notes from this application to a CSV file that follows the formatting required for importing calendar files to Google Calendar.
The exporting process can be invoked by the user with the following command:
Command: note export fn/FILE_NAME
-
The command
note export
will create a file with a .csv extension on the local storage. * -
Invoking the command above by itself will convert all exportable notes data saved in the application to CSV format.
-
[Coming in V2.0] It can be extended to perform more specific instructions. The following optional arguments may be used:
-
note export fn/FILE_NAME [fr/START_DATE to/END_DATE]
The following command allows the user to export notes from a specific date range.
-
Implementation of exporting to CSV is provided below.
1. Assuming that exportable notes data are currently present in Trajectory, the user can invoke the note export
command to begin exporting.
2. For each Note object to be exported, a corresponding CsvAdaptedNote object will be created. It is used to generate the correct formatting for the Google Calendar.
3. A CSV utility class will then be used to handle the writing to CSV. The file is saved to "/data/CSVexport" folder in the application’s directory.
The following sequence diagram shows the note exporting process:
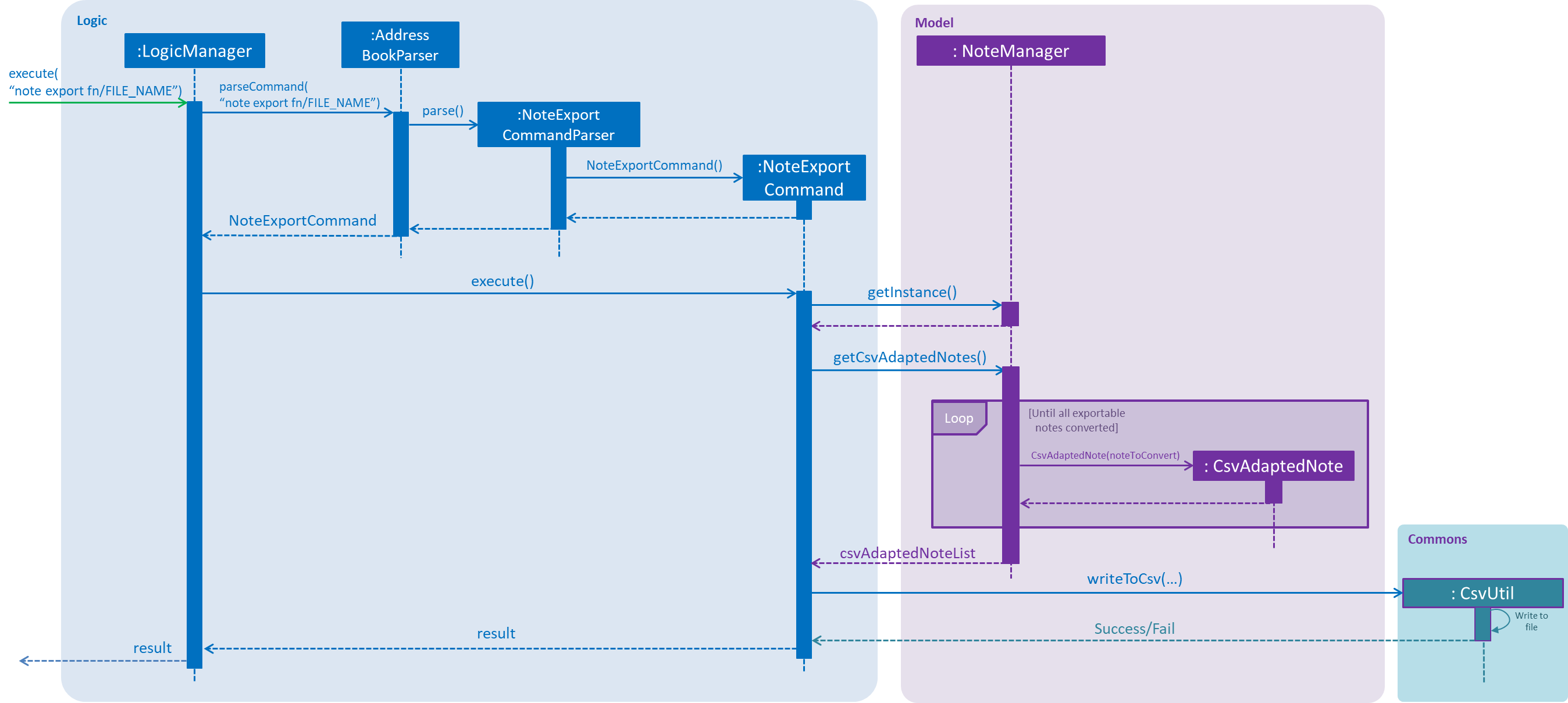
3.8. Logging
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Section 3.9, “Configuration”) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
3.9. Configuration
Certain properties of the application can be controlled (e.g App name, logging level) through the configuration file (default: config.json
).
4. Documentation
We use asciidoc for writing documentation.
We chose asciidoc over Markdown because asciidoc, although a bit more complex than Markdown, provides more flexibility in formatting. |
4.1. Editing Documentation
See UsingGradle.adoc to learn how to render .adoc
files locally to preview the end result of your edits.
Alternatively, you can download the AsciiDoc plugin for IntelliJ, which allows you to preview the changes you have made to your .adoc
files in real-time.
4.2. Publishing Documentation
See UsingTravis.adoc to learn how to deploy GitHub Pages using Travis.
4.3. Converting Documentation to PDF format
We use Google Chrome for converting documentation to PDF format, as Chrome’s PDF engine preserves hyperlinks used in webpages.
Here are the steps to convert the project documentation files to PDF format.
-
Follow the instructions in UsingGradle.adoc to convert the AsciiDoc files in the
docs/
directory to HTML format. -
Go to your generated HTML files in the
build/docs
folder, right click on them and selectOpen with
→Google Chrome
. -
Within Chrome, click on the
Print
option in Chrome’s menu. -
Set the destination to
Save as PDF
, then clickSave
to save a copy of the file in PDF format. For best results, use the settings indicated in the screenshot below.
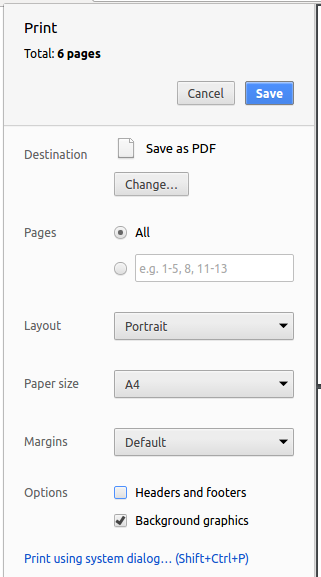
4.4. Site-wide Documentation Settings
The build.gradle
file specifies some project-specific asciidoc attributes which affects how all documentation files within this project are rendered.
Attributes left unset in the build.gradle file will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
The name of the website. If set, the name will be displayed near the top of the page. |
not set |
|
URL to the site’s repository on GitHub. Setting this will add a "View on GitHub" link in the navigation bar. |
not set |
|
Define this attribute if the project is an official SE-EDU project. This will render the SE-EDU navigation bar at the top of the page, and add some SE-EDU-specific navigation items. |
not set |
4.5. Per-file Documentation Settings
Each .adoc
file may also specify some file-specific asciidoc attributes which affects how the file is rendered.
Asciidoctor’s built-in attributes may be specified and used as well.
Attributes left unset in .adoc files will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
Site section that the document belongs to.
This will cause the associated item in the navigation bar to be highlighted.
One of: * Official SE-EDU projects only |
not set |
|
Set this attribute to remove the site navigation bar. |
not set |
4.6. Site Template
The files in docs/stylesheets
are the CSS stylesheets of the site.
You can modify them to change some properties of the site’s design.
The files in docs/templates
controls the rendering of .adoc
files into HTML5.
These template files are written in a mixture of Ruby and Slim.
Modifying the template files in |
5. Testing
5.1. Running Tests
There are three ways to run tests.
The most reliable way to run tests is the 3rd one. The first two methods might fail some GUI tests due to platform/resolution-specific idiosyncrasies. |
Method 1: Using IntelliJ JUnit test runner
-
To run all tests, right-click on the
src/test/java
folder and chooseRun 'All Tests'
-
To run a subset of tests, you can right-click on a test package, test class, or a test and choose
Run 'ABC'
Method 2: Using Gradle
-
Open a console and run the command
gradlew clean allTests
(Mac/Linux:./gradlew clean allTests
)
See UsingGradle.adoc for more info on how to run tests using Gradle. |
Method 3: Using Gradle (headless)
Thanks to the TestFX library we use, our GUI tests can be run in the headless mode. In the headless mode, GUI tests do not show up on the screen. That means the developer can do other things on the Computer while the tests are running.
To run tests in headless mode, open a console and run the command gradlew clean headless allTests
(Mac/Linux: ./gradlew clean headless allTests
)
5.2. Types of tests
We have two types of tests:
-
GUI Tests - These are tests involving the GUI. They include,
-
System Tests that test the entire App by simulating user actions on the GUI. These are in the
systemtests
package. -
Unit tests that test the individual components. These are in
seedu.address.ui
package.
-
-
Non-GUI Tests - These are tests not involving the GUI. They include,
-
Unit tests targeting the lowest level methods/classes.
e.g.seedu.address.commons.StringUtilTest
-
Integration tests that are checking the integration of multiple code units (those code units are assumed to be working).
e.g.seedu.address.storage.StorageManagerTest
-
Hybrids of unit and integration tests. These test are checking multiple code units as well as how the are connected together.
e.g.seedu.address.logic.LogicManagerTest
-
5.3. Troubleshooting Testing
Problem: HelpWindowTest
fails with a NullPointerException
.
-
Reason: One of its dependencies,
HelpWindow.html
insrc/main/resources/docs
is missing. -
Solution: Execute Gradle task
processResources
.
6. Dev Ops
6.1. Build Automation
See UsingGradle.adoc to learn how to use Gradle for build automation.
6.2. Continuous Integration
We use Travis CI and AppVeyor to perform Continuous Integration on our projects. See UsingTravis.adoc and UsingAppVeyor.adoc for more details.
6.3. Coverage Reporting
We use Coveralls to track the code coverage of our projects. See UsingCoveralls.adoc for more details.
6.4. Documentation Previews
When a pull request has changes to asciidoc files, you can use Netlify to see a preview of how the HTML version of those asciidoc files will look like when the pull request is merged. See UsingNetlify.adoc for more details.
6.5. Making a Release
Here are the steps to create a new release.
-
Update the version number in
MainApp.java
. -
Generate a JAR file using Gradle.
-
Tag the repo with the version number. e.g.
v0.1
-
Create a new release using GitHub and upload the JAR file you created.
6.6. Managing Dependencies
A project often depends on third-party libraries. For example, Address Book depends on the Jackson library for XML parsing. Managing these dependencies can be automated using Gradle. For example, Gradle can download the dependencies automatically, which is better than these alternatives.
a. Include those libraries in the repo (this bloats the repo size)
b. Require developers to download those libraries manually (this creates extra work for developers)
7. Product Scope
Target user profile:
-
faculty members of any education institutions
-
has a need to manage a significant number of students
-
prefer desktop apps over other types of applications (mobile, web-based)
-
can type fast
-
prefers typing over mouse input
-
is reasonably comfortable using CLI apps
Value proposition: manage students faster than a typical mouse/GUI driven app
Appendix A: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
teacher |
add students |
keep track of students that are currently in the institution |
|
teacher |
remove students |
remove students who have graduated or are no longer with the institution |
|
teacher |
find students |
get relevant student details, such as contact information |
|
teacher |
list students |
look at all the students that are currently in the institution |
|
teacher |
import students |
import students from perhaps an existing LMS solution |
|
teacher |
export students |
have a copy of my students data set for possible import into another system |
|
teacher |
add course |
assign students to courses and keep track of who is in which course |
|
teacher |
delete course |
delete courses that may no longer be in use |
|
teacher |
list courses |
view all courses that exist within the institution |
|
teacher |
list student list by course |
view all students taking a certain course so I can plan my module enrollment better |
|
teacher |
add modules |
manage my module matters more easily |
|
teacher |
update modules |
change the details of my modules after I have created them |
|
teacher |
remove modules |
delete modules that I accidentally created |
|
teacher |
view module details |
see extra information such as the students enrolled in the module |
|
teacher |
archive modules |
remove modules that I am no longer actively teaching, and keep it as a historical record instead |
|
teacher |
find modules |
check if I have already created the module, and view its details if it exists in the system |
|
teacher |
list modules |
see all the modules I am currently managing |
|
teacher |
enrol students in a module |
keep track of the students taking my various modules |
|
teacher |
assign a TA |
get assistance in managing the module |
|
teacher |
create a class |
assign students to the class |
|
teacher |
list a class |
display information of the class |
|
teacher |
delete a class |
remove a class that is created wrongly |
|
teacher |
assign student to class |
add students to a class in the event that some students still have not signed up for a slot when classes begin |
|
teacher |
unassign student from class |
remove a student from a class if the student has dropped out of school, or if he/she has not paid his/her school fees |
|
teacher |
modify class enrollment limit |
set class enrollment limits so that the classes that I’m teaching or my TAs are teaching are not over-subscribed. |
|
teacher |
access class attendance list |
access and view the class attendance to see which students are present/absent |
|
teacher |
mark class attendance list |
mark the attendance for every present student |
|
teacher |
modify class attendance list |
alter a wrongly-marked attendance for a specific student |
|
teacher |
add gradebook components |
differentiate the grade components in a module (E.g. mid term test, finals examination) |
|
teacher |
edit gradebook components |
modifications is possible to reflect the grade component properly. |
|
teacher |
delete gradebook components |
incorrect grade components in the module can be removed. |
|
teacher |
list gradebook components |
view the grade components available in the module. |
|
teacher |
find gradebook components |
view the information for a specific grade component. |
|
teacher |
add students grades |
store the marks for students enrolled in module |
|
teacher |
list students grades |
view all grades keyed in to the students |
|
teacher |
display students grades in a graph |
keep track of the progress of students for a particular grade component. |
|
teacher |
add notes |
keep track of important things and also my own teaching progress |
|
teacher |
delete notes |
remove completed tasks or discard those that are no longer needed |
|
teacher |
view saved notes |
easily check up on important things I could have forgotten |
|
teacher |
edit notes from modules |
efficiently make changes to my notes if needed without deleting and then adding a new one |
|
teacher |
assign priorities to notes |
make effective planning by looking for notes with higher importance |
|
teacher |
attach deadlines to notes |
keep track of upcoming deadlines and important dates |
|
teacher |
find specific notes |
search for notes quickly without having to go through an entire list |
|
teacher |
lock the system |
be sure that no one can see confidential student data when I’m away |
|
teacher |
unlock the system |
continue to use the system when I’m back from a break after having previously locked it |
Appendix B: Use Cases
(For all use cases below, the System is Trajectory
and the Actor is the teacher
, unless specified otherwise)
Use case: Unlock system
Precondition(s) :
-
The system must be locked.
Guarantees :
-
NIL
MSS :
-
Teacher enters password.
-
System verifies that the password is correct, and displays a message to that effect.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters improperly formatted command.
-
2b1. System displays the proper format for usage of the command.
Use case resumes at step 1.
-
-
2c. Invalid password.
-
2c1. System shows 'wrong password' message.
Use case resumes at step 1.
-
Use case: Lock system
Precondition(s) :
-
NIL
Guarantees :
-
NIL
MSS :
-
Teacher enters command.
-
System locks the system, and displays a message to that effect.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters improperly formatted command.
-
2b1. System displays the proper format for usage of the command.
Use case resumes at step 1.
-
Use case: Add Student
Precondition(s) :
-
NIL
Guarantees :
-
NIL
MSS :
-
Teacher adds student to system.
-
System adds student to system, and show a confirmation message.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters improperly formatted command.
-
2b1. System displays the proper format for usage of the command.
Use case resumes at step 1.
-
-
2c. Student already exists in system.
-
2c1. System shows 'duplicate student' message.
Use case resumes at step 1.
-
Use case: Remove Student
Precondition(s) :
-
Student must exist in the system.
Guarantees :
-
NIL
MSS :
-
Teacher removes student from system.
-
System removes student to system, and show a confirmation message.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters improperly formatted command.
-
2b1. System displays the proper format for usage of the command.
Use case resumes at step 1.
-
-
2c. Student not found in system.
-
2c1. System shows 'invalid student' message.
Use case resumes at step 1.
-
Use case: Find Student
Precondition(s) :
-
NIL
Guarantees :
-
NIL
MSS :
-
Teacher finds student with entered details.
-
System locates student details and displays it to the teacher.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters improperly formatted command.
-
2b1. System displays the proper format for usage of the command.
Use case resumes at step 1.
-
-
2c. Student not found in system.
-
2c1. System shows 'invalid student' message.
Use case resumes at step 1.
-
Use case: Delete Student
Precondition(s) :
-
NIL
Guarantees :
-
NIL
MSS :
-
Teacher deletes student by index.
-
System deletes the student from the system, and shows a confirmation message.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters improperly formatted command.
-
2b1. System displays the proper format for usage of the command.
Use case resumes at step 1.
-
-
2c. Invalid index.
-
2c1. System shows 'invalid index' message.
Use case resumes at step 1.
-
Use case: List Students
Precondition(s) :
-
NIL
Guarantees :
-
NIL
MSS :
-
Teacher lists students.
-
System displays list of all students by default.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters improperly formatted command.
-
2b1. System displays the proper format for usage of the command.
Use case resumes at step 1.
-
-
2c. There are no students in the system.
-
2c1. System shows 'no students in system' message.
Use case resumes at step 1.
-
Use case: Export All Students To File [COMING IN V2.0]
Precondition(s) :
-
There should be at least 1 student.
Guarantees :
-
NIL
MSS :
-
Teacher exports all students to file.
-
System exports all students to file and display confirmation message.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters improperly formatted command.
-
2b1. System displays the proper format for usage of the command.
Use case resumes at step 1.
-
-
2c. Invalid save location.
-
2c1. System shows 'invalid save location' message.
Use case resumes at step 1.
-
-
2d. No students to export.
-
2d1. System shows 'no students to export' message.
Use case resumes at step 1.
-
Use case: Import students from file [COMING IN V2.0]
Precondition(s) :
-
A file containing properly formatted data should exist.
Guarantees :
-
NIL
MSS :
-
Teacher imports students from file.
-
System imports students from file and display confirmation message.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters improperly formatted command.
-
2b1. System displays the proper format for usage of the command.
Use case resumes at step 1.
-
-
2c. Invalid file location.
-
2c1. System shows 'invalid file location' message.
Use case resumes at step 1.
-
-
2d. File in invalid format.
-
2d1. System shows 'invalid file format' message.
Use case resumes at step 1.
-
Use case: Add course
Precondition(s) :
-
NIL
Guarantees :
-
NIL
MSS :
-
Teacher adds course.
-
System adds course to system, and show a confirmation message.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters improperly formatted command.
-
2b1. System displays the proper format for usage of the command.
Use case resumes at step 1.
-
-
2c. Course already exists in system.
-
2c1. System shows 'duplicate course' message.
Use case resumes at step 1.
-
Use case: List courses
Precondition(s) :
-
NIL
Guarantees :
-
NIL
MSS :
-
Teacher lists courses.
-
System displays list of all courses.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters improperly formatted command.
-
2b1. System displays the proper format for usage of the command.
Use case resumes at step 1.
-
Use case: Remove course
Precondition(s) :
-
NIL
Guarantees :
-
NIL
MSS :
-
Teacher removes course.
-
System removes course from the system, and show a confirmation message.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters improperly formatted command.
-
2b1. System displays the proper format for usage of the command.
Use case resumes at step 1.
-
-
2c. Invalid course code
-
2c1. System shows 'invalid course code' message.
Use case resumes at step 1.
-
-
2d. There are students assigned to that course code.
-
2d1. System shows 'no deletion for course that has registered students' message.
Use case resumes at step 1.
-
Use case: Edit course
Precondition(s) :
-
NIL
Guarantees :
-
NIL
MSS :
-
Teacher edits a course with specified details.
-
System edits course details, and shows a confirmation message.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters improperly formatted command.
-
2b1. System displays the proper format for usage of the command.
Use case resumes at step 1.
-
-
2c. Course does not exist in system.
-
2c1. System shows 'invalid course' message.
Use case resumes at step 1.
-
Use case: List courses ordered by students
Precondition(s) :
-
NIL
Guarantees :
-
NIL
MSS :
-
Teacher lists courses ordered by students.
-
System displays lists of courses ordered by students.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters improperly formatted command.
-
2b1. System displays the proper format for usage of the command.
Use case resumes at step 1.
-
Use case: Add module
Precondition(s) :
-
NIL
Guarantees :
-
No duplicate modules will be allowed.
MSS :
-
Teacher wants to add a module to the system.
-
System successfully adds the module.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters the wrong parameter prefix.
-
2b1. System displays the correct format for the command.
Use case resumes at step 1.
-
-
2c. Teacher enters a module code that already exists in the system.
-
2c1. System informs the user of the existence of the module.
Use case resumes at step 1.
-
-
2d. Teacher fills in the prerequisites with module codes that don’t exist.
-
2d1. System informs the user of the non-existing module codes.
Use case resumes at step 1.
-
Use case: Update module
Precondition(s) :
-
The module should already exist in Trajectory.
Guarantees :
-
NIL
MSS :
-
Teacher wants to edit a module to the system.
-
System successfully saves the changes made to the module.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters the wrong parameter prefix.
-
2b1. System displays the correct format for the command.
Use case resumes at step 1.
-
-
2c. Teacher enters a module code that doesn’t exist in the system.
-
2c1. System informs the user that the module doesn’t exist.
Use case resumes at step 1.
-
-
2d. Teacher fills in the prerequisites with module codes that don’t exist.
-
2d1. System informs the user of the non-existing module codes.
Use case resumes at step 1.
-
Use case: Remove module
Precondition(s) :
-
The module should already exist in Trajectory.
Guarantees :
-
NIL
MSS :
-
Teacher wants to delete a module in the system.
-
System prompts for confirmation to delete the module.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters the wrong parameter prefix.
-
2b1. System displays the correct format for the command.
Use case resumes at step 1.
-
-
2c. Teacher enters a module code that doesn’t exist in the system.
-
2c1. System informs the user that the module doesn’t exist.
Use case resumes at step 1.
-
Use case: View module details
Precondition(s) :
-
The module should already exist in Trajectory.
Guarantees :
-
NIL
MSS :
-
Teacher wants to see the details of a particular module in the system.
-
System displays all the information about that module.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the command’s correct usage.
Use case resumes at step 1.
-
Use case: Find module
Precondition(s) :
-
At least one module should exist in Trajectory.
Guarantees :
-
NIL
MSS :
-
Teacher searches for a module with some module codes as keywords.
-
System lists all the active modules that match any of the keywords.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters keywords that do not match any modules.
-
2b1. System informs the user that no active modules were found.
Use case resumes at step 1.
-
Use case: List modules
Precondition(s) :
-
At least one module should exist in Trajectory.
Guarantees :
-
NIL
MSS :
-
Teacher wants to see all the active modules in the system.
-
System lists all the active modules.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
Use case: Enrol students in modules
Precondition(s) :
-
The module should already exist in Trajectory.
-
The student(s) should exist in Trajectory.
Guarantees :
-
NIL
MSS :
-
Teacher wants to enrol students in a module.
-
System successfully enrols the students in the module.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters the wrong parameter prefix.
-
2b1. System displays the correct format for the command.
Use case resumes at step 1.
-
-
2c. Teacher enters the wrong email format.
-
2c1. System displays the correct format for the command.
Use case resumes at step 1.
-
-
2d. Teacher enters the command without any matric no. and emails.
-
2d1. System displays the correct format for the command.
Use case resumes at step 1.
-
-
2e. Teacher enters a module code that doesn’t exist in the system.
-
2e1. System informs the user that the module doesn’t exist in the system.
Use case resumes at step 1.
-
-
2f. Teacher wants to enrol a student that doesn’t exist in the system.
-
2f1. System informs the user that the student doesn’t exist in the system.
Use case resumes at step 1.
-
Use case: Archive module [coming in v2.0]
Precondition(s) :
-
The module should already exist in Trajectory.
Guarantees :
-
NIL
MSS :
-
Teacher wants to archive a module in the system.
-
System prompts for confirmation to archive the module.
-
Teacher confirms archiving of the module.
-
System successfully archives the module.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters the wrong parameter prefix.
-
2b1. System displays the correct format for the command.
Use case resumes at step 1.
-
-
2c. Teacher enters a module code that doesn’t exist in the system.
-
2c1. System informs the user that the module doesn’t exist.
Use case resumes at step 1.
-
-
3a. Teacher rejects the confirmation to delete the module.
Use case resumes at step 1.
Use case: Assign a TA [coming in v2.0]
Precondition(s) :
-
The module should already exist in Trajectory.
-
The student(s) should exist in Trajectory.
Guarantees :
-
NIL
MSS :
-
Teacher assigns a student as a TA of a module.
-
System successfully assigns the student as a TA for the module.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters the wrong parameter prefix.
-
2b1. System displays the correct format for the command.
Use case resumes at step 1.
-
-
2c. Teacher enters the wrong email format.
-
2c1. System displays the correct format for the command.
Use case resumes at step 1.
-
-
2d. Teacher enters the command without any matric no. and emails.
-
2d1. System displays the correct format for the command.
Use case resumes at step 1.
-
-
2e. Teacher enters a module code that doesn’t exist in the system.
-
2e1. System informs the user that the module doesn’t exist in the system.
Use case resumes at step 1.
-
-
2f. Teacher wants to enrol a student that doesn’t exist in the system.
-
2f1. System informs the user that the student doesn’t exist in the system.
Use case resumes at step 1.
-
Use case: Add Gradebook Component
Precondition(s) :
-
Gradebook component name NOT exist in existing module.
-
Accumulated weightage for gradebook components in module cannot exceed 100%.
Guarantees : NIL
MSS :
-
Teacher creates gradebook component.
-
System indicates success message.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. Teacher enters the wrong parameter prefix.
-
2b1. System displays the correct format for the command.
Use case resumes at step 1.
-
Use case: Update Gradebook Component
Precondition(s) :
-
Gradebook component name must exist in existing module.
-
Accumulated weightage including the updated weightage must not exceed 100%.
-
At least one optional parameter must be included in command.
Guarantees : NIL
MSS :
-
Teacher updates gradebook component.
-
System indicates success message.
Use case ends.
Extensions :
-
1a. System detects an error in the entered data.
-
1a1. System displays message and format corresponding to error.
-
1a2. Teacher enters new data.
Steps 1a1-1a2 are repeated until the data entered is correct.
Use case resumes from step 1.
-
Use case: Remove Gradebook Component
Precondition(s) :
-
Gradebook component name must exist in existing module.
Guarantees :
-
Deleting gradebook component will also delete any student marks associated to it.
MSS :
-
Teacher removes gradebook component.
-
System indicates success message.
Use case ends.
Extensions :
-
2a. Teacher enters an invalid command.
-
2a1. System displays the list of valid commands.
Use case resumes at step 1.
-
Use case: Find Gradebook Component
Precondition(s) :
-
Gradebook component name must exist in existing module.
Guarantees : NIL
MSS :
-
Teacher finds gradebook component.
-
System displays details on selected gradebook component.
Use case ends.
Extensions :
-
1a. Teacher enters an invalid command.
-
1a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
1b. Teacher enters the wrong parameter prefix.
-
1b1. System displays the correct format for the command.
Use case resumes at step 1.
-
Use case: List Gradebook Components
Precondition(s) :
-
Gradebook component name must exist in existing module.
Guarantees : NIL
MSS :
-
Teacher lists gradebook component.
-
System displays list.
Use case ends.
Extensions :
-
1a. System detects an error in the entered data.
-
1a1. System displays message and format corresponding to error.
Use case resumes from step 1.
-
Use case: List Students Grades
Precondition(s) :
-
Students must be enrolled to the module.
Guarantees : NIL
MSS :
-
Teacher lists students grades.
-
System displays list.
Use case ends.
Extensions :
-
1a. System detects an error in the entered data.
-
1a1. System displays message and format corresponding to error.
-
1a2. Teacher enters new data.
Steps 1a1-1a2 are repeated until the data entered is correct.
Use case resumes from step 1.
-
Use case: Assign Student Grade
Precondition(s) :
-
Students must be enrolled to the module.
-
Grade component name must exist in existing module.
-
Marks assigned to student for the particular gradebook component must not exceed its maximum marks.
Guarantees : NIL
MSS :
-
Teacher assigns student a mark.
-
System indicates success message.
Use case ends.
Extensions :
-
1a. System detects an error in the entered data.
-
1a1. System displays message and format corresponding to error.
-
1a2. Teacher enters new data.
Steps 1a1-1a2 are repeated until the data entered is correct.
Use case resumes from step 1.
-
Use case: Display Graph of Student Grades
Precondition(s) :
-
Grade component name must exist in existing module.
-
Marks of all students taking the module should be added in.
Guarantees : NIL
MSS :
-
Teacher displays grade graph.
-
System indicates success message.
Use case ends.
Extensions :
-
1a. System detects an error in the entered data.
-
1a1. System displays message and format corresponding to error.
-
1a2. Teacher enters new data.
Steps 1a1-1a2 are repeated until the data entered is correct.
Use case resumes from step 1.
-
Use case: Add Class
Precondition(s) :
-
Module code exists in data file.
Guarantees :
-
NIL
MSS :
-
User enters command to create classroom.
-
Classroom is created for the module.
-
System displays message of successful creation of class.
Use case ends.
Extensions :
-
1a. User entered invalid command.
-
1a1. System shows ‘invalid format’ error.
Use case resumes at step 1.
-
Use case: List Class
Precondition(s) :
-
Class(es) exists in data file.
Guarantees :
-
NIL
MSS :
-
User enters command to list class(es).
-
All Classroom information is listed.
-
System displays message of successful listing of class(es).
Use case ends.
Extensions :
-
1a. User entered invalid command.
-
1a1. System shows ‘invalid format’ error.
Use case resumes at step 1.
-
Use case: Update Class Enrollment Limits
Precondition(s) :
-
Module code exists in data file.
-
Class belonging to the module code exists in data file.
Guarantees :
-
NIL
MSS :
-
User enters command to modify class enrollment limits.
-
Class enrollment limits gets updated.
-
System displays successful modification of class enrollment limits.
Use case ends.
Extensions :
-
1a. User entered invalid command.
-
1a1. System shows ‘invalid format’ error.
Use case resumes at step 1.
-
Use case: Remove Class
Precondition(s) :
-
Module code exists in the data file.
-
Class belonging to the module code exists in data file.
Guarantees :
-
NIL
MSS :
-
User enters command to delete a class from module.
-
Classroom is deleted from module.
-
System displays message of successful deletion of class from module.
Use case ends.
Extensions :
-
1a. User entered invalid command.
-
1a1. System shows ‘invalid format’ error.
Use case resumes at step 1.
-
-
1b. Specified class does not belong to module.
-
1b1. System displays specified class does not belong to module error.
Use case resumes at step 1.
-
Use case: Assign Student To Class
Precondition(s) :
-
Module code exists in data file.
-
Student exists and enrolled into module in data file.
-
Class must not be full.
-
Class belonging to the module code exists in data file.
Guarantees :
-
NIL
MSS :
-
User enters command to assign a student to class.
-
Student gets assigned to class.
-
System displays message of successful assignment of student to class.
Use case ends.
Extensions :
-
1a. User entered invalid command.
-
1a1. System shows ‘invalid format’ error.
Use case resumes at step 1.
-
-
1b. Class doesn’t exist.
-
1b1. System displays class not found error.
Use case resumes at step 1.
-
Use case: Unassign Student From Class
Precondition(s) :
-
Module code exists in data file.
-
Student exists and enrolled into module in data file.
-
Class belonging to the module code exists in data file.
-
Student must be assigned to class before unassigning them.
Guarantees :
-
NIL
MSS :
-
User enters command to unassign a student from class.
-
Student gets unassigned from class.
-
System displays message of successful unassignment of student from class.
Use case ends.
Extensions :
-
1a. User entered invalid command.
-
1a1. System shows ‘invalid format’ error.
Use case resumes at step 1.
-
-
1b. Module code doesn’t exist.
-
1b1. System displays module not found error.
Use case resumes at step 1.
-
-
1c. Specified student does not belong to class.
-
1c1. System displays specified student does not belong to class error.
Use case resumes at step 1.
-
Use case: Mark Class Attendance List
Precondition(s) :
-
Student exists in data file.
-
Module code exists in data file.
-
Class belonging to module code exists in data file.
-
Student must be assigned to class.
Guarantees :
-
NIL
MSS :
-
User enters command to mark class attendance.
-
Class attendance is marked for specified student.
-
System displays message of successful marking of class attendance list.
Use case ends.
Extensions :
-
1a. User entered invalid command.
-
1a1. System shows ‘invalid format’ error.
Use case resumes at step 1.
-
-
1b. Specified student does not belong to class.
-
1b1. System displays specified student does not belong to class error.
Use case resumes at step 1.
-
Use case: Access Class Attendance List
Precondition(s) :
-
Module code exists in data file.
-
Class belonging to module code exists in data file.
Guarantees :
-
NIL
MSS :
-
User enters command to view class attendance list.
-
System displays the class attendance list.
Use case ends.
Extensions :
-
1a. User entered invalid command.
-
1a1. System shows ‘invalid format’ error.
Use case resumes at step 1.
-
Use case: Modify Class Attendance List
Precondition(s) :
-
Module code exists in data file.
-
Class belonging to the module code exists in data file.
-
Student exists in data file and must be marked present.
Guarantees :
-
NIL
MSS :
-
User enters command to modify class attendance list.
-
The class attendance list is updated.
-
System displays message of successful modification of class attendance list.
Use case ends.
Extensions :
-
1a. User entered invalid command.
-
1a1. System shows ‘invalid format’ error.
Use case resumes at step 1.
-
Use case: Add Note
Precondition(s)
-
Module must exist in data file.
Guarantees :
-
NIL
MSS :
-
Teacher requests to add a note.
-
System prompts the teacher to enter his/her note.
-
Teacher types the note.
-
System adds the note to the module and displays a message that it is successfully added.
Use case ends.
Extensions :
-
1a. Teacher enters an invalid command.
-
1a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
1b. The module code specified does not exist in Trajectory.
-
1b1. System informs user that module does not exist.
Use case resumes at step 1.
-
-
3a. The teacher decides to cancel.
Use case ends.
Use case: List Notes
Precondition(s) :
-
Notes must exist in data file.
Guarantees :
-
NIL
MSS :
-
Teacher requests to view the saved notes.
-
System displays the complete numbered list of notes.
Use case ends.
Extensions :
-
1a. Teacher enters an invalid command.
-
1a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. There are no saved entries of notes.
-
2b1. System displays a message that no entries are found.
Use case ends.
-
Use case: Edit Note
Precondition(s) :
-
Note must exist in data file.
-
Module must exist in data file.
Guarantees :
-
NIL
MSS :
-
Teacher requests to list all notes.
-
System displays the complete numbered list of notes.
-
Teacher requests to edit a specific note in the list.
-
System prompts the teacher to enter the modifications.
-
Teacher can now modify the text.
-
System saves the modified note and displays a message for the successful modification.
Use case ends.
Extensions :
-
1a. Teacher gives an invalid command.
-
1a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2a. There are no saved entries of notes.
-
2a1. System displays a message that no entries are found.
Use case ends.
-
-
3a. The given index is invalid.
-
3a1. System informs the user that the input is invalid.
Use case resumes at step 2.
-
-
3b. The module code specified does not exist in Trajectory.
-
3b1. System informs user that module does not exist.
Use case resumes at step 3.
-
-
5a. The teacher decides to cancel.
-
5a1. System cancels the editing process.
Use case ends.
-
Use case: Delete Notes
Precondition(s) :
-
Note must exist in data file.
Guarantees :
-
NIL
MSS :
-
Teacher requests to list all notes.
-
System displays the complete numbered list of notes.
-
Teacher requests to delete one or more notes in the list.
-
System deletes the note(s) and displays a message for successful deletion.
Use case ends.
Extensions :
-
1a. Teacher enters an invalid command.
-
1a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2a. There are no saved entries of notes in the module.
-
2a1. System displays a message that no entries are found.
Use case ends.
-
-
3a. Teacher enters an invalid command.
-
3a1. System displays the list of valid commands.
Use case resumes at step 3.
-
-
3b. The given index is invalid.
-
3b1. System informs the user that the input is invalid.
Use case resumes at step 2.
-
Use case: Find Notes
Precondition(s) :
-
Notes must exist in data file.
Guarantees :
-
NIL
MSS :
-
Teacher requests to find notes which contains a set of keywords.
-
System displays the complete numbered list of notes found that contains the keyword(s).
Use case ends.
Extensions :
-
1a. Teacher enters an invalid command.
-
1a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. There are no entries found that contains the keyword(s).
-
2b1. System displays a message that no entries are found.
Use case ends.
-
Use case: Export Notes
Precondition(s) :
-
Notes must exist in data file.
-
Notes must contain a start date and end date.
Guarantees :
-
NIL
MSS :
-
Teacher requests to export notes.
-
System informs the user the number of notes exported.
Use case ends.
Extensions :
-
1a. Teacher enters an invalid command.
-
1a1. System displays the list of valid commands.
Use case resumes at step 1.
-
-
2b. There are no notes that can be exported.
-
2b1. System displays a message that no entries are found.
Use case ends.
-
Appendix C: Non Functional Requirements
-
Privacy
-
Students’ and faculty members' private contact details shouldn’t be disseminated without prior consent.
-
-
Data Retention
-
User data shouldn’t be retained after a certain amount of time after a student graduates to protect their personal data.
-
-
Cross-platform
-
Should work on any mainstream OS as long as it has Java
9
or higher installed.
-
-
Responsiveness
-
Should be able to hold up to 1000 persons without a noticeable sluggishness in performance for typical usage.
-
-
Ease of Use
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
-
Appendix E: Product Survey
E.1. Moodle
Author: Martin Dougiamas and the Moodle Community.
Pros:
-
Free, Open Source
-
Widely-used
Cons:
-
Fairly complex.
-
Requires some set up, and there’s no support for offline use.
E.2. Integrated Virtual Learning Environment (IVLE)
Author: NUS CIT
Pros:
-
Built to serve the NUS community specifically.
Cons:
-
Closed-source.
-
No support for offline use.
Appendix F: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
F.1. Launch and Shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
F.2. Lock/Unlock
-
Unlocking access to Trajectory
-
Prerequisites: The system must be locked.
-
Test case:
unlock pw/password
Expected: Correct password. You’ll gain access to the system, and be able to use commands right away. -
Test case:
unlock pw/password2
Expected: Wrong password. You’ll get an error, and you won’t be able to use any other commands.
-
-
Relocking access to Trajectory
-
Prerequisites: The system must be unlocked.
-
Test case:
lock
Expected: The system will be locked. You will be barred from typing any other commands until you re-lock the system.
-
F.3. Course Management
-
Adding a course
-
Prerequisites: The course must not already exist.
-
Test case:
course add c/CEG n/Computer Engineering f/School of Computing
Expected: This course will be added into the system.
-
-
Listing a course
-
Prerequisites: NONE.
-
Test case:
course list
Expected: The list of courses in the system will be displayed. If there are none, the output should be blank.
-
-
Listing students by courses
-
Prerequisites: It’s better to run this command when you’ve added students to registered courses.
-
Test case:
course liststudents
Expected: The list of students ordered by course in the system will be displayed. If there are none, the output should be blank.
-
-
Deleting a course
-
Prerequisites: The course code 'CEG' must exist.
-
Test case:
course delete c/CEG
Expected: This course will be removed from the system.
-
-
Editing a course
-
Prerequisites: The course code 'CEG' must exist.
-
Test case:
course edit c/CEG n/Comp Eng f/FOE
Expected: This course will be modified to the specified details.
-
F.4. Student Management
-
Adding a student
-
Prerequisites: The student must not already exist, and the course code must have been added.
-
Test case:
student add n/Megan Nicole i/A0168000B c/CEG p/98765432 e/johnd@example.com a/311, Clementi Ave 2, #02-25
Expected: This student will be added into the system. -
Test case:
student add n/Taylor Swift i/A0160B c/CEG p/98765432 e/taylorswift@ts.com a/311, Clementi Ave 3, #03-25
Expected: The matric. no is of an invalid form. You won’t be allowed to add this student.
-
-
Listing students
-
Prerequisites: NONE.
-
Test case:
student list
Expected: The list of students in the system will be displayed. If there are none, the output should be blank.
-
-
Finding a student
-
Prerequisites: There should be a student with the name "Megan Nicole".
-
Test case:
student find Megan
Expected: The list of students with the name "Megan" will be displayed.
-
-
Editing a student
-
Prerequisites: There should be a student with the name "Megan Nicole".
-
Test case:
student edit n/Megan Nicole c/EEE
Expected: The student will have her course code changed to EEE.
-
-
Removing a student
-
Prerequisites: There should be at least one student in the system. Run 'student list' first.
-
Test case:
student delete 1
Expected: The student at INDEX 1 will be deleted from the system.
-
F.5. Module Management
-
Adding a module
-
Prerequisites: The module must not already exist.
-
Test case:
module add mc/NM2212 mn/Visual Design
Expected: This module will be added into the system. -
Test case:
module add mc/NNNNNN mn/Visual Design
Expected: The module code is not valid. You will not be able to add the module. -
Test case:
module add mc/NM2212 mn/Visual!@@$%#
Expected: The module name contains special characters that are not allowed. You will not be able to add the module.
-
-
Editing a module
-
Prerequisites: There must be a module with code NM2212.
-
Test case:
module edit mc/NM2212 mn/VD
Expected: The module name will be changed to VD.
-
-
Deleting a module
-
Prerequisites: There must be a module with code NM2212.
-
Test case:
module delete mc/NM2212
Expected: The module name will be changed to VD. -
Test case:
module delete mc/NE1000 mn/Non-existent module
Expected: The module with code NE1000 cannot be found. You won’t be able to delete it.
-
-
Listing modules
-
Prerequisites: NONE.
-
Test case:
module list
Expected: The list of modules in the system will be displayed. If there are no modules, the output should be blank.
-
-
Finding a module
-
Prerequisites: There should be a module with the word
Design
, either in the module code or module name. -
Test case:
module find design
Expected: The list of modules with the wordDesign
in the name will be displayed.
-
-
Enrolling a student into a module
-
Prerequisites: There should be a student with matric no. A0168000B, and a module with module code NM2212.
-
Test case:
module enrol mc/NM2212 i/A0168000B
Expected: The student with matric no. A0168000B will be enrolled in the module. The module details will be displayed.
-
-
Viewing a module’s details
-
Prerequisites: There should be a module with module code NM2212.
-
Test case:
module view mc/NM2212
Expected: The module details for NM2212 will be displayed, including the list of students enrolled in the module.
-
F.6. Creating a class
-
Creates a class and assigns it to a module for the system.
Parameters (prefix):
cn/CLASS_NAME,
mc/MODULE_CODE,
e/MAX_ENROLLMENT
-
Prerequisites: Module code must exist before creating a class for the module.
-
Test case:
class add cn/T16 mc/CS2113 e/20
Expected: A new class T16 of module code CS2113 and enrollment size of 20 will be created and reflected in the system. -
Test case:
class add cn/T16 mc/CS2113 e/9999
Expected: A message will be displayed to show that enrollment size should be of 1-425 and it should not be blank. -
Test case:
class add cn/T1600 mc/CS2113 e/20
Expected: A message will be displayed to show that class name should only contain between 1 to 3 alphanumeric characters and it should not be blank. -
Other incorrect class add commands to try:
class add
,
class add cn/T16 mc/XXYYYY e/20
(where XXYYYY is a valid module code that does not exist),
class add cn/VVV mc/ZZXXXX e/20
(where VVV and ZZXXXX is the classname and module code of a class already added into the system)
Expected: A message will be displayed to showInvalid command format!
,
Module code does not exist
,
andThis classroom already exists in Trajectory
respectively.
F.7. Listing a class
-
Lists class(es) with information of the class as well as students assigned to class (if any) for the system.
Parameters (prefix): NIL
-
Prerequisites: Class(es) must exist in order to be listed.
-
Test case:
class list
Expected: The class information is display on the system.
F.8. Update Class Enrollment Limits
-
Modifies the max enrollment size for a class for the system.
Parameters (prefix):
cn/CLASS_NAME,
mc/MODULE_CODE,
e/ENROLLMENT_SIZE
-
Prerequisites: Module code must exist before deleting a class for the module. The classroom must also belong to the module code.
-
Test case:
class edit cn/T16 mc/CS2113 e/69
Expected: The max enrollment size of class T16 of module code CS2113 will be updated to 69 and reflected in the system. -
Test case:
class edit cn/T16 mc/CS2113 e/9999
Expected: A message will be displayed to show that enrollment size should be of 1-425 and it should not be blank. -
Other incorrect class edit commands to try:
class edit
,
class edit cn/T16 mc/XXYYYY e/20
(where XXYYYY is a valid module code that does not exist),
class edit cn/VVV mc/XXYYYY e/20
(where VVV is a valid class name that don’t belong to existing module XXYYYY)
Expected: A message will be displayed to showInvalid command format!
,
Module code does not exist
,
andClass belonging to module not found!
respectively.
F.9. Deleting a class
-
Deletes a class for a module in the system.
Parameters (prefix):
cn/CLASS_NAME,
mc/MODULE_CODE
-
Prerequisites: Module code must exist before deleting a class for the module. The classroom must also belong to the module code.
-
Test case:
class delete cn/T16 mc/CS2113
Expected: The class T16 of module code CS2113 deleted and reflected in the system. -
Other incorrect class delete commands to try:
class delete
,
class delete cn/VVV mc/XXYYYY
(where VVV is a valid class name not belonging to a valid module XXYYYY)
Expected: A message will be displayed to showInvalid command format!
,
andClass belonging to module not found!
respectively.
F.10. Assign Student To Class
-
Assigns a student to a class in the system.
Parameters (prefix):
cn/CLASS_NAME,
mc/MODULE_CODE,
i/MATRIC_NO
-
Prerequisites: Student must exist in the system, module code must exist, student must be enrolled in the module, class cannot be full and class must exist and belong to the module code before assigning a student to the class.
-
Test case:
class addstudent cn/T16 mc/CS2113 i/A0168372L
Expected: A new student of matric number A0168372L will be assigned to the classroom T16 of module CS2113 and be reflected in the system. -
Other incorrect class addstudent commands to try:
class addstudent
,
class addstudent cn/VVV mc/ZZZZZZ i/XXXXXXXXX
(where XXXXXXXXX is a valid matric number that does not exist, VVV and ZZZZZZ is a valid existing class),
class addstudent cn/VVV mc/ZZZZZZ i/XXXXXXXXX
(where XXXXXXXXX is an existing student matric number enrolled into ZZZZZZ, VVV is a valid class name format but do not belong to an existing module ZZZZZZ),
class addstudent cn/VVV mc/ZZZZZZ i/XXXXXXXXX
(where the student of matric number XXXXXXXXX is already assigned to the existing classroom comprised of VVV and ZZZZZZ),
Expected: A message will be displayed to showInvalid command format!
,
Student does not exist
,
Class belonging to module not found!
,
This student already exists in class: XXXXXXXXX
(where XXXXXXXXX is the matric number of the student already in the classroom) respectively.
F.11. Unassign Student From Class
-
Unassigns a student to a class in the system.
Parameters (prefix):
cn/CLASS_NAME,
mc/MODULE_CODE,
i/MATRIC_NO
-
Prerequisites: Student must exist in the system, module code must exist, student must be enrolled in the module, class must exist and belong to the module code and student must be assigned to the class before unassigning a student from the class.
-
Test case:
class delstudent cn/T16 mc/CS2113 i/A0168372L
Expected: The student of matric number A0168372L will be unassigned from the classroom T16 of module CS2113 and be reflected in the system. -
Other incorrect class delstudent commands to try:
class delstudent
,
class delstudent cn/VVV mc/ZZZZZZ i/XXXXXXXXX
(where XXXXXXXXX is a valid matric number format that does not exist, VVV and ZZZZZZ is a valid existing class),
class delstudent cn/VVV mc/ZZZZZZ i/XXXXXXXXX
(where XXXXXXXXX is an existing student matric number enrolled into ZZZZZZ, VVV is a valid class name not belonging to an existing module ZZZZZZ),
class delstudent cn/VVV mc/ZZZZZZ i/XXXXXXXXX
(where the student of matric number XXXXXXXXX is already unassigned from the existing classroom comprised of VVV and ZZZZZZ),
Expected: A message will be displayed to showInvalid command format!
,
Student does not exist
,
Class belonging to module not found!
,
This student doesn’t belong to class: XXXXXXXXX
(where XXXXXXXXX is the matric number of the student not in the classroom) respectively.
F.12. Marking class attendance list
-
Mark the class attendance list for a specified student for the system.
Parameters (prefix):
cn/CLASS_NAME,
mc/MODULE_CODE,
i/MATRIC_NO
-
Prerequisites: Student must exist in the system, module code must exist, class must exist and belong to the module code, student must belong to class before marking the student present for the class.
-
Test case:
class markattendance cn/T16 mc/CS2113 i/A0168372L
Expected: The student of matric number A0168372L from the classroom T16 of module CS2113 will be marked present and be reflected in the system. -
Other incorrect class markattendance commands to try:
class markattendance
,
class markattendance cn/VVV mc/ZZZZZZ i/XXXXXXXXX
(where XXXXXXXXX is a valid matric number format but does not exist, VVV and ZZZZZZ is a valid existing class),
class markattendance cn/VVV mc/ZZZZZZ i/XXXXXXXXX
(where XXXXXXXXX is an existing student matric number enrolled into ZZZZZZ, VVV is a valid class name not belonging to an existing module ZZZZZZ),
class markattendance cn/VVV mc/ZZZZZZ i/XXXXXXXXX
(where the student of matric number XXXXXXXXX is already marked present in the existing classroom comprised of VVV and ZZZZZZ),
Expected: A message will be displayed to showInvalid command format!
,
Student does not exist
,
Class belonging to module not found!
,
This student already present in class: XXXXXXXXX
(where XXXXXXXXX is the matric number of the student already marked present) respectively.
F.13. Access class attendance list
-
Access the class attendance list for the system.
Parameters (prefix):
cn/CLASS_NAME,
mc/MODULE_CODE
-
Prerequisites: Module must exist, class must exist and belong to the module code, at least one student’s attendance is marked before being able to be listed.
-
Test case:
class listattendance cn/T16 mc/CS2113
Expected: The class attendance list for the class T16 of module CS2113 will be shown (if attendance is taken) and be reflected in the system. -
Other incorrect class listattendance commands to try:
class listattendance
,
class listattendance cn/VVV mc/ZZZZZZ
(where VVV is a valid class name and ZZZZZZ is a valid non-existant module),
class listattendance cn/VVV mc/ZZZZZZ
(where VVV is a valid class name not belonging to an existing module ZZZZZZ),
Expected: A message will be displayed to showInvalid command format!
,
Module code does not exist
,
Class belonging to module not found!
respectively.
F.14. Modify class attendance list
-
Modifies the class attendance list for the system.
Parameters (prefix):
cn/CLASS_NAME,
mc/MODULE_CODE,
i/MATRIC_NO
-
Prerequisites: Module must exist, class must exist and belong to the module code, student must exist and belong to the class and marked present before being able to modify their attendance to absent.
-
Test case:
class modattendance cn/T16 mc/CS2113 i/A0168372L
Expected: The student of matric number A0168372L from the classroom T16 of module CS2113 will be marked absent and be reflected in the system. -
Other incorrect class modattendance commands to try:
class modattendance
,
class modattendance cn/VVV mc/ZZZZZZ i/XXXXXXXXX
(where XXXXXXXXX is a valid matric number that does not exist, VVV and ZZZZZZ is a valid existing class),
class modattendance cn/VVV mc/ZZZZZZ i/XXXXXXXXX
(where XXXXXXXXX is an existing student matric number enrolled into ZZZZZZ, VVV is a valid class name not belonging to an existing module ZZZZZZ),
class modattendance cn/VVV mc/ZZZZZZ i/XXXXXXXXX
(where the student of matric number XXXXXXXXX is already marked absent in the existing classroom comprised of VVV and ZZZZZZ),
Expected: A message will be displayed to showInvalid command format!
,
Student does not exist
,
Class belonging to module not found!
,
This student’s attendance is already absent: XXXXXXXXX
(where XXXXXXXXX is the matric number of the student already marked absent) respectively.
F.15. Gradebook List
-
Listing all gradebook components to existing module(s) in Trajectory.
Parameters (prefix): NIL-
Prerequisites: NIL
-
Test case:
gradebook list
Expected: Gradebook list will be displayed on the system with all the gradebook components set to a module. The total number of gradebook components are indicated in the status message.
-
F.16. Gradebook Add
-
Adding a gradebook component to an existing module in Trajectory.
Parameters (prefix):
Module code (mc/),
gradebook component name (cn/),
[optional] maximum marks (mm/),
[optional] weightage(w/).-
Prerequisites: Module code must already exist in system. Gradebook component name cannot already exist to module. In this case, take 'CS2113' as a module that already exist in Trajectory.
-
Test case:
gradebook add mc/CS2113 cn/Assignment 1
Expected: Gradebook list will be displayed on the system with the newly added gradebook component 'Assignment 1'. The total number of gradebook components are indicated in the status message. -
Test case:
gradebook add mc/CS2113 cn/Assignment 1 mm/20
Expected: Gradebook component will not be added into the system as 'Assignment 1' is already found in the system. Error details shown in the status message. -
Other incorrect gradebook add commands to try:
gradebook add
,
gradebook add mc/CS2113 cn/x mm/y
(where x is not an existing component name to 'CS2113', y is a value below 0 and above 100),
gradebook add mc/CS2113 cn/x w/y
(where x is not an existing component name to 'CS2113', y is a value below 0 and above 100),
gradebook add mc/CS2113 cn/x w/y
(where x is not an existing component name to 'CS2113', where y is a value above the remaining weightage available to module code → Add up all weightage of grade components set to 'CS2113''),
Expected: Similar to previous.
-
F.17. Gradebook Find
-
Finding a gradebook component to an existing module in Trajectory.
Parameters (prefix):
Module code (mc/),
gradebook component name (cn/).-
Prerequisites: Gradebook component name to a module must already exist in system. In this case, take 'Assignment 1' as the gradebook component set to 'CS2113' in Trajectory.
-
Test case:
gradebook find mc/CS2113 cn/Assignment 1
Expected: Information of the gradebook component to module will be displayed on the system. -
Test case:
gradebook find mc/CS2113 cn/Finals
Expected: Since gradebook component is not found in the system, error details will be shown in the status message. -
Other incorrect gradebook find commands to try:
gradebook find
,
gradebook find mc/MA1511 cn/Finals
Expected: Similar to previous.
-
F.18. Gradebook Delete
-
Deleting a gradebook component to an existing module in Trajectory.
Parameters (prefix):
Module code (mc/),
gradebook component name (cn/).-
Prerequisites: Gradebook component name to a module must already exist in system. In this case, take 'Assignment 1' as only the gradebook component set to 'CS2113' in Trajectory.
-
Test case:
gradebook delete mc/CS2113 cn/Assignment 1
Expected: Gradebook list will be displayed on the system with the deleted gradebook component missing from the list. The total number of gradebook components remaining are indicated in the status message. -
Test case:
gradebook delete mc/CS2113 cn/Finals
Expected: Since gradebook component is not found in the system, error details will be shown in the status message. -
Other incorrect gradebook delete commands to try:
gradebook delete
,
gradebook delete mc/MA1511 cn/Finals
Expected: Similar to previous.
-
F.19. Gradebook Edit
-
Editing a gradebook component to an existing module in Trajectory.
Parameters (prefix):
Module code (mc/),
gradebook component name (cn/),
[Optional] edited gradebook component name (en/),
[Optional] edited maximum marks (mm/),
[Optional] edited weightage [w/].
*Take note that at least one optional parameter must be indicated in the command.-
Prerequisites: Gradebook component name to a module must already exist in system. In this case, take 'Assignment 1' as the gradebook components set to 'CS2113' in Trajectory.
-
Test case:
gradebook edit mc/CS2113 cn/Assignment 1 mm/20
Expected: Gradebook list will be displayed on the system with the edited gradebook component. The total number of gradebook components is indicated in the status message. -
Test case:
gradebook edit mc/CS2113 cn/Finals w/50
Expected: Since gradebook component is not found in the system, error details will be shown in the status message. -
Other incorrect gradebook edit commands to try:
gradebook edit
,
gradebook edit mc/CS2113 cn/Assignment 1 w/x
where x is a value above the remaining weightage available to module code → Add up all weightage of grade components set to 'CS2113'
Expected: Similar to previous. === Grade Add
-
-
Adding marks to a student for grade component to a module. In this case, take 'Assignment 1' with maximum marks of 20 as the gradebook components set to 'CS2113' in Trajectory. Student enrolled to the module has matric no. 'A0169999A'
Parameters (prefix):
Module code (mc/),
Gradebook component name (cn/),
Student Matric Number (i/),
Marks (m/).-
Prerequisites: Gradebook component name to a module must already exist in system. Student must be enrolled to the module.
-
Test case:
grade add mc/CS2113 cn/Assignment 1 i/A0169999A m/15
Expected: Grade list will be displayed. The total number of gradebook components is indicated in the status message. -
Test case:
grade add mc/CS2113 cn/Assignment 1 i/A0169999A m/30
Expected: Since the marks indicated is above the maximum marks set of 20, error details will be shown in the status message. -
Other incorrect grade add commands to try:
grade add
,
grade add mc/CS2113 cn/Assignment 1 i/x m/10
where x is a matric number of a student that is not enrolled in module.
Expected: Similar to previous.
-
F.20. Grade List
-
Listing all grade of students of an existing gradebook component for module in Trajectory.
Parameters (prefix): NIL-
Prerequisites: NIL
-
Test case:
grade list
Expected: Grade list will be displayed on the system with all the grade information. The total number of grade components remaining are indicated in the status message.
-
F.21. Grade Graph
-
Displaying the graph of all students marks to a gradebook component for a module in Trajectory. In this case, take 'Assignment 1' as the gradebook components set to 'CS2113' in Trajectory. Alse, all marks of students enrolled to the module is already in the system.
Parameters (prefix):
Module code (mc/),
Gradebook component name (cn/).-
Prerequisites: All students enrolled in the module must have their marks recorded. Else, user has no access to graph.
-
Test case:
grade graph mc/CS2113 cn/Assignment 1
Expected: Grade graph will be pop up. -
Other incorrect grade graph commands to try:
grade graph
,
grade add mc/CS2113 cn/x
where x is a grade component is either not associated to module or not all marks of students enrolled in module is recorded in Trajectory.
Expected: Similar to previous.
-
F.22. Adding a note
-
Adding a note in Trajectory while all notes are listed.
Parameters (prefix):
[optional] MODULE_CODE (mc/),
[optional] NOTE_TITLE (tt/),
[optional] NOTE_START_DATE (sd/),
[optional] NOTE_START_TIME (st/),
[optional] NOTE_END_DATE (ed/),
[optional] NOTE_END_TIME (et/),
[optional] NOTE_LOCATION (lc/)-
Prerequisites: List all notes using
note list
command. The displayed list can have 0 or more notes. MODULE_CODE must already exist in the system (if specified as parameter). Take 'CS2113' as a module that already exists in Trajectory. -
Test case:
note add
Expected: The application will prompt the user to enter note. Upon saving, the notes list should be displayed with the newly created note at the bottom. If cancelled, the note should not be saved. -
Test case:
note add tt/First note sd/16-11-2018 lc/NUS
Expected: Similar to previous, but the new note should contain more information when displayed. -
Test case:
note add mc/CS2113
Expected: Similar to previous. However, this note will be assigned to 'CS2113' module. -
Test case:
note add mc/GEQ1000
Expected: No note is added. An error message will be shown. -
Other incorrect add commands to try:
note add tt/x
(where x is a string of characters with character count greater than 30)
Expected: Same as previous.
-
F.23. Deleting notes
-
Deleting notes in Trajectory while all notes are listed.
Parameters (prefix):
INDEX (no prefix),
INDEX_RANGE (no prefix)-
Prerequisites: List all notes using
note list
command. The displayed list contains multiple notes. -
Test case:
note delete 1
Expected: The first note labeled '#1' in the list will be deleted. A message will be displayed upon successful deletion. -
Test case:
note delete 1-3
Expected: The notes #1, #2, and #3 will be deleted from the list. A message will be displayed upon successful deletion. -
Test case:
note delete 0
Expected: No note is deleted. An error message will be shown. -
Other incorrect delete commands to try:
note delete -1
,note delete 3-1
,note delete x
(where x is larger than the list size)
Expected: Same as previous.
-
F.24. Editing a note
-
Editing a note in Trajectory while all notes are listed.
Parameters (prefix):
INDEX (no prefix),
[optional] MODULE_CODE (mc/),
[optional] NOTE_TITLE (tt/),
[optional] NOTE_START_DATE (sd/),
[optional] NOTE_START_TIME (st/),
[optional] NOTE_END_DATE (ed/),
[optional] NOTE_END_TIME (et/),
[optional] NOTE_LOCATION (lc/)-
Prerequisites: List all notes using
note list
command. The displayed list contains multiple notes. MODULE_CODE must already exist in the system (if specified as parameter). Take 'CS2113' as a module that already exists in Trajectory. -
Test case:
note edit 1
Expected: The application will prompt the user to modify the note. Upon saving, the list will be updated. If cancelled, the modifications are discarded. -
Test case:
note edit 1 tt/Modified Title
Expected: Same as previous but the title of the note will also be edited. -
Test case:
note edit x
(where x is larger than the list size)
Expected: No note is edited. An error message will be shown.
-
F.25. Listing notes
-
Listing all existing notes in Trajectory.
Parameters (prefix):
[optional] MODULE_CODE (mc/)-
Prerequisites: Multiples notes are currently saved in Trajectory. At least one note should be assigned to 'CS2113'. Take 'CS2113' as a module that already exists in Trajectory.
-
Test case:
note list
Expected: The list of all notes in Trajectory will be displayed. -
Test case:
note list mc/CS2113
Expected: The list of all notes assigned to 'CS2113' module will be displayed. -
Test case:
note list mc/GER1000
Expected: A message indicating that no notes were found should be displayed. The list will not be updated.
-
F.26. Finding notes by keywords
-
Finding notes in Trajectory that matches keyword(s).
Parameters (prefix):
KEYWORD (k/),
[optional] MORE_KEYWORDS (k/)-
Prerequisites: Multiples notes are currently saved in Trajectory. At least one note should contain the word "hello" in its title or in the text of the note.
-
Test case:
note find k/hello
Expected: The list of all notes containing the word "hello" will be displayed. -
Test case:
note find k/x
(where x is a word that does not exist in any note)
Expected: A message indicating that no notes were found should be displayed. The list will not be updated.
-
F.27. Exporting notes to CSV
-
Exporting notes in Trajectory.
Parameters (prefix):
FILE_NAME (fn/)-
Prerequisites: Multiples notes are currently saved in Trajectory. At least one note should have a date & time displayed in its information when
note list
command is used. -
Test case:
note export fn/test_case1
Expected: A message indicating a successful export will be displayed. A file named "test_case1.csv" will be saved in /data/CSVexport/ directory from Trajectory’s location. -
Test case:
note export fn/test_case@
Expected: No notes will be exported and no file is created. An error message indicating an invalid filename will be displayed.
-